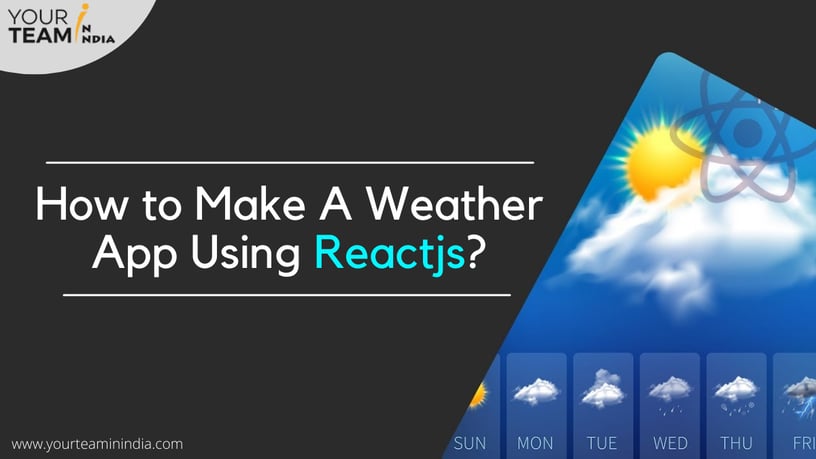
Note: This blog was originally published on 20th October 2021 and updated on 16th March 2023.
Quick Summary: The blog "Build a Weather App in ReactJS" provides a step-by-step guide to create a weather app using ReactJS and Open Weather Map API. The author starts by explaining the importance of having a weather app and then dives into setting up the environment, installing dependencies, and creating components for the app. The article also covers API integration and styling the app using Bootstrap. Overall, the blog provides a useful tutorial for beginners who want to build a simple weather app using ReactJS.
So, without further ado, let’s get started!
In today's world, weather apps have become an integral part of our daily lives. They provide us with important information about the weather, which can be used to plan our activities accordingly. In this context, building a weather app using ReactJS can be a great learning experience for beginners in the field of web development. The blog "Build a Weather App in ReactJS" provides a detailed guide on how to create a simple yet functional weather app using ReactJS and OpenWeatherMap API. It covers all the essential aspects of building a weather app, from setting up the environment to integrating API and styling the app.
Key Takeaways
- ReactJS is a popular JavaScript library for building user interfaces.
- The OpenWeatherMap API can be integrated into a weather app built using ReactJS.
- Styling a React app can be done using various tools and libraries.
- Deploying a React app requires creating a production build and hosting it on a web server or cloud platform.
- Integrating the OpenWeatherMap API requires making HTTP requests and handling responses.
Features of the Weather App
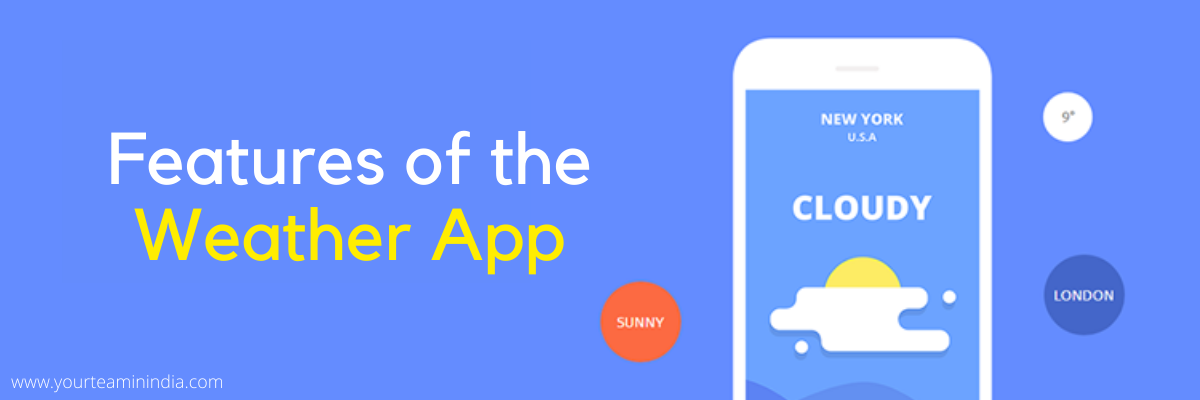
✅ Display the five-day weather forecast for a specific location
✅ Allow app users to toggle between Celsius and Fahrenheit
✅ Display the weather icon, description of the weather, and temperature reading
Also, the app will rely on the following to be absolutely reliable and scalable.
- Create React App
- Open Weather Map API
- Open Weather Font
- Moment.js
- Bootstrap 4
Note: In order to continue, you will need to set it up all.
Recommended Read: 15+ Top React Libraries to Try
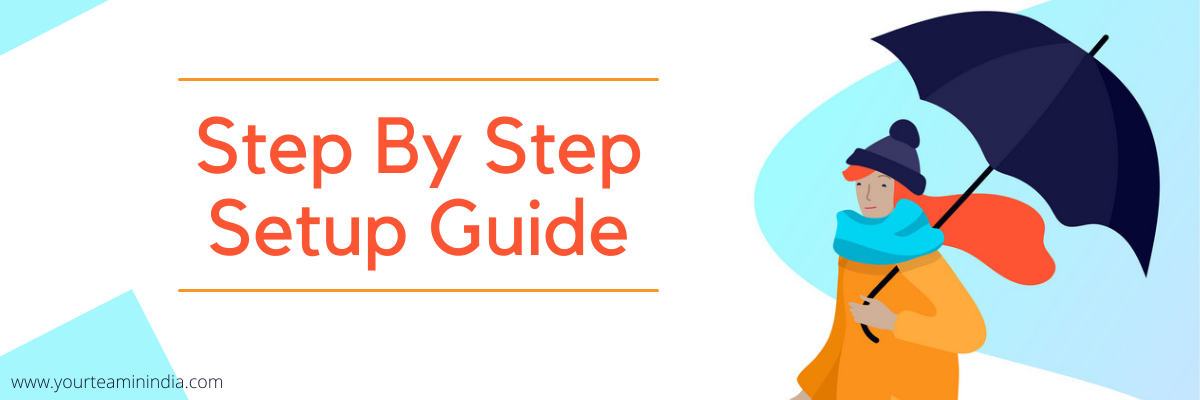
The Initial Stage
You will need to run this code in the terminal:
$ create-react-app new-weather-app
Once that is done, you will need to run two lines in the terminal:
$ cd new-weather-app
$ npm start
It will let you see the React app update live on a localhost server. After that, you will need to open the project, delete the default contents that App.js comes with, and then create a new file in the src folder called WeekContainer.js. After that, import this class component into App.js and add it to the render method of the App.
File Path: WeekContainer.js
import React from 'react';
class WeekContainer extends React.Component {
render() {
return (
Hello World!
)
}
}
export default WeekContainer;
File Path: App.js
import React, { Component } from 'react';
import './App.css';
import WeekContainer from './WeekContainer';
class App extends Component {
render() {
return (
);
}
}
export default App;
Weather Data for WeekContainer
In this step, we will acquire the weather data for WeekContainer, which might be rendering properly onscreen. Now, it is time to add some information. The container’s responsibility is to hold five weather cards, with each one representing a day of the week.
For that, we need to fetch the data from the API. Here we have used OpenWeatherMap API.
- How to Get and Hide an API Key?
- Sign up for an account on the website
- Once you do that, an email is received having an API key to activate within a few hours.
- Now create a file within the src folder and add your API key to it.
- After that, it is time to add this file to the .gitignore file so that when pushing to GitHub, this file is not considered, rather ignored.
- Once that is done, import the hidden file within the WeekContainer. Now, to use your API key in the fetch request, you will need to use the string interpolation,
So, after you have completed all these steps, the WeekContainer will appear something like this on the screen:
import React from 'react';
import apiConfig from './apiKeys';
class WeekContainer extends React.Component {
render() {
const weatherURL =
`http://api.openweathermap.org/data/2.5/forecast?zip=11102&units=imperial&APPID=${apiConfig.owmKey}`
return (
Hello World!
)
}
}
export default WeekContainer;
Fetch from the Open Weather Map API
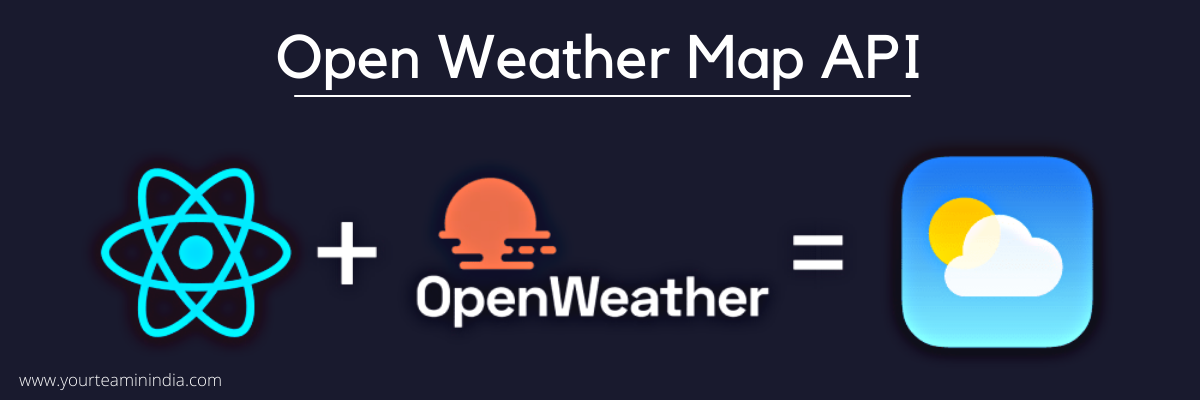
So once you have access to the API, you will have to fetch it after that. For that, create a ComponentDidMount method and then include the code given below:
componentDidMount = () => {
const weatherURL =
`http://api.openweathermap.org/data/2.5/forecast?zip=11102&units=imperial&APPID=${apiConfig.owmKey}`
fetch(weatherURL)
.then(res => res.json())
.then(data => console.log("Data List Loaded", data.list))
}
With this code, you have fetched the data from API using the API key, parsed it to json, and printed it to the console to check how successful that was.
Recommended Read: 15 Advantages of ReactJS for Application Development
Now, Filtration
The WeekContainer stores five cards, with each representing every day of the week. We need to filter the readings just to show one reading every day. Also, keep in mind that the data is an object and to get data.list, you will need different objects, with every object representing the weather reading.
const dailyData = data.list.filter(reading => {
return reading.dt_txt.includes("18:00:00")
}
)
To read the object, we will use dt_txt, which is a string version of the date. Check the condole.log, which must look like this:
“2019–02–28 03:00:00”
The time will be in relation to the UTC rather than your local timezone.
Also, the filter needs to give only five readings as objects:
import React from 'react';
import apiConfig from './apiKeys';
class WeekContainer extends React.Component {
state = {
fullData: [],
dailyData: []
}
componentDidMount = () => {
const weatherURL =
`http://api.openweathermap.org/data/2.5/forecast?zip=11102&units=imperial&APPID=${apiConfig.owmKey}`
fetch(weatherURL)
.then(res => res.json())
.then(data => {
const dailyData = data.list.filter(reading => reading.dt_txt.includes("18:00:00"))
this.setState({
fullData: data.list,
dailyData: dailyData
}, () => console.log(this.state))
})
}
render() {
return (
Hello World!
)
}
}
export default WeekContainer;
Let’s Render Cards for Every Day
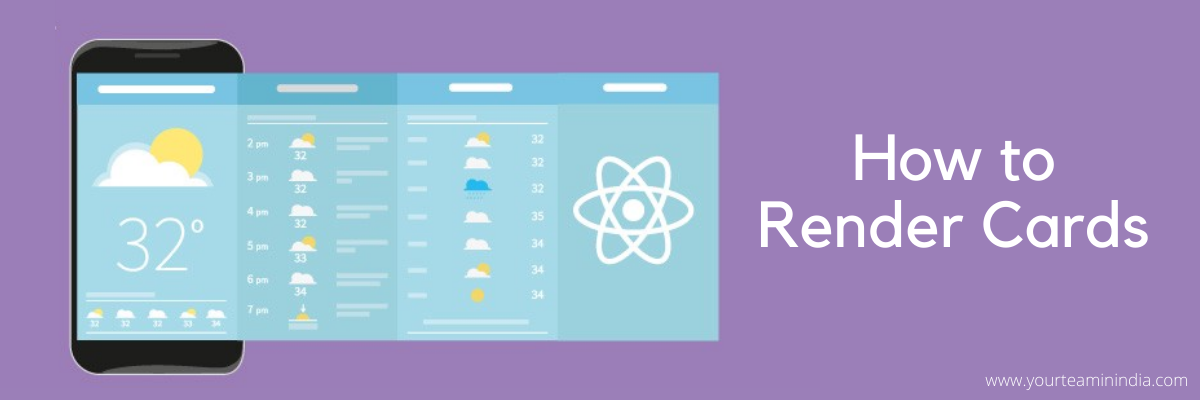
We will map the different objects in WeekContainer of DayCard components and directly render that array. So you will need to import the DayCard component in WeekContainer. Also, we will need to make DayCard’s a functional component. For that, we will create the function in the WeekContainer, responsible for rendering DayCards. Create a new function, return the map, and call a function in the render. Use this.functionName. You can even utilize the map within the render method before saving it into a variable and calling it in return.
formatDayCards = () => {
return this.state.dailyData.map((reading, index) => )
}
render() {
return (
{this.formatDayCards()}
)
}
We have the WeekContainer holding five DayCard components ready, and now we must work on displaying the relevant information based on every card. Also, we willneed to intsall Bootstrap 4 to convert our cards into soemthing that is easily distinguishable.
Adding Bootstrap 4
Run this code in your terminal:
$ npm install bootstrap
You will have to add this code to the head of your HTML document, index.html in the public folder.
After that, you can use the Bootstrap in the WeekContainer, you will have to give the main div a class of container and div holding the DayCards a class of row justidy-content-center. It will display like this:
render() {
return (
5-Day Forecast.
New York, US
{this.formatDayCards()}
)
}
Adding Information to the Card
We will convert the data into the human-readable format as Open Weather Map will give us majorly the cryptic data.
- Day of the Week
We need to input the code given below to return the day of the week using the Moment.js.
let newDate = new Date();
const weekday = reading.dt * 1000
newDate.setTime(weekday)
...
moment(newDate).format(“dddd”)
Use this code to show the date and time:
moment(newDate).format(“MMMM Do, h:mm a”)
Using OpenWeatherFont Icons
For this step, we need to ensure that the owfonts are installed. For these icons to appear, it is required to make a string interpolation.
`owf owf-${props.reading.weather[0].id} owf-5x`
Temperature
Reading.main.temp is the reading of the temperature and you will have to round and append “ °F” to the end of it.
Weather Condition Description
Type reading.weather[0].description to access the weather’s description.
The Card.js must look like this:
import React from 'react';
var moment = require('moment');
const DayCard = ({ reading }) => {
let newDate = new Date();
const weekday = reading.dt * 1000
newDate.setTime(weekday)
const imgURL = `owf owf-${reading.weather[0].id} owf-5x`
return (
{moment(newDate).format('dddd')}
{moment(newDate).format('MMMM Do, h:mm a')}
{Math.round(reading.main.temp)} °F
{reading.weather[0].description}
)
}
export default DayCard;
Fahrenheit and Celsius Toggle
The app looks fine, however to make it more interactive, here’s what you need to do. At this point, you have your API call hardcorded to ask for readings to be in Fahrenheit. To change it into celsius, we need to change units=imperial to units=metric. Hence, we have to render a toggle switch so as to interact with the users. Here’s how you can do it:
- Send a new fetch request to the API with the units parameter altered
- Now, we will use state in WeekContainer to send props to the DayCards.
Adding Degree Toggle
We will make the DegreeToggle component, which will render the toggle switch. Here we will make DegreeToggle a functional component. We will use the radio buttons and here is what you code should appear like:
import React from 'react';
const DegreeToggle = props => {
return (
<input
class="form-check-input"
type="radio"
name="degree-type"
id="celsius"
value="metric"
/>
<input
class="form-check-input"
type="radio"
name="degree-type"
id="farenheit"
value="imperial"
/>
)
}
export default DegreeToggle;
Connecting DegreeToggle to the WeekContainer
The Child component of WeekContainer is DegreeToggle, which is to be imported into WeekContainer. Create a function and pass it down as a prop to DeegreeToggle in render method.
WeekContainer- State Usage
After everything is wired together, we will then implement the functionality. Also we need to keep track of DayCards to render with Celsisus or Fahrenheit. We can do that by saving a key called degreeType into our state and passing the information along to our DayCards. We will add the DegreeType to State and pass DegreeToggel as a prop with the function responsible to update degreeType.
state = {
fullData: [],
dailyData: [],
degreeType: "fahrenheit"
}
updateForecastDegree = event => {
this.setState({
degreeType: event.target.value
}, () => console.log(this.state))
}
Now we will update radio buttons to invoke the callback prop given to them from WeekContainer onChange and have their values reflect the degreeType stored in the WeekContainer. We even call it a controlled component.
import React from 'react';
const DegreeToggle = ({degreeType, updateForecastDegree}) => {
return (
<input
className="form-check-input"
type="radio"
name="degree-type"
id="celsius"
value="celsius"
checked={degreeType === "celsius"}
onChange={updateForecastDegree}
/>
<input
className="form-check-input"
type="radio"
name="degree-type"
id="farenheit"
value="fahrenheit"
checked={degreeType === "fahrenheit"}
onChange={updateForecastDegree}
/>
)
}
export default DegreeToggle;
DayCards Render Celsius
Pass degreeType to DayCards to render them in Celsisus for the ‘celsius’ degreeType. So, for this you will need to pass degreeType as another prop to DayCards. After that is done, create a variable that hold the celsius value converted from Fahrenheit value. Finally, you will need to add a conditional into card’s portion where the degree is rendered.
import React from 'react';
var moment = require('moment');
const DayCard = ({ reading, degreeType }) => {
let newDate = new Date();
const weekday = reading.dt * 1000
newDate.setTime(weekday)
const fahrenheit = Math.round(reading.main.temp)
const celsius = Math.round((fahrenheit - 32) * 5/9)
const imgURL = `owf owf-${reading.weather[0].id} owf-5x`
return (
{moment(newDate).format('dddd')}
{moment(newDate).format('MMMM Do, h:mm a')}
{degreeType === "celsius" ? celsius + "°C" : fahrenheit + "°F"}
{reading.weather[0].description}
)
}
export default DayCard;
Conclusion
Voila! You have got a highly reliable and functional weather app ready using ReactJS. Also, if you are running a business, you can rely on an experienced ReactJS developer to create such a highly reliable app for you. Go ahead and try it yourself to see how it works.
Need help setting up a dedicated team of Reactjs developers in India? At Your Team In India, we have a pool of certified Reactjs engineers. Connect with us our business head now and get a free consultation.
Frequently Asked Questions [FAQs]
Q: Which is the best API for weather?
There are many weather APIs available, and the best one depends on the specific needs of your project. Some of the most popular weather APIs are:
-
OpenWeatherMap
- Weather Underground
- AccuWeather
- Dark Sky
- Weatherbit
- Climacell
Q: Is weather API a REST API?
Yes, weather APIs can be implemented as REST APIs. REST (Representational State Transfer) is a commonly used architectural style for web services, where the client and server communicate through HTTP requests and responses. Weather APIs can expose RESTful endpoints that allow clients to retrieve weather data using HTTP GET requests.
Q: Is Google weather API free?
Sign up for a free account now and immediately begin using our weather API to query accurate forecast & historical data for any global location.