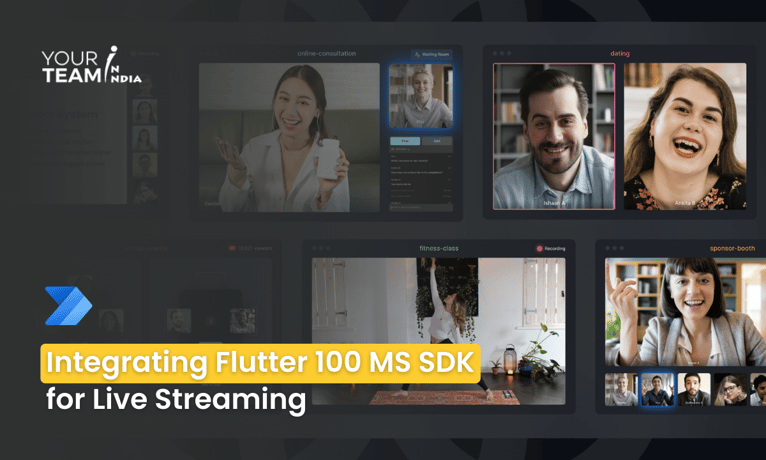
Quick Summary: Dive into the world of live streaming in Flutter with the 100 MS SDK integration. This article serves as your comprehensive guide to seamlessly incorporating the 100 MS SDK into your Flutter applications, enabling real-time video streaming capabilities for a dynamic user experience.
Introduction
In the realm of mobile app development, Flutter has gained immense popularity due to its cross-platform capabilities and a rich set of widgets. For developers looking to integrate live streaming into their Flutter applications, the 100 MS SDK provides a robust solution. This article will guide you through the process of integrating the 100 MS SDK into a Flutter app and implementing live-streaming features.
Prerequisites
Before you begin, make sure you have the following prerequisites:
- Flutter SDK is installed on your development machine.
- An IDE such as Visual Studio Code or Android Studio.
- A 100 MS developer account and API key.
Step 1: Create a new Flutter project
Open your terminal and run the following command to create a new Flutter project:
flutter create flutter_live_streaming cd flutter_live_streaming |
Step 2: Add 100 MS SDK to dependencies
Open the `pubspec.yaml` file in your project and add the 100 MS SDK dependency:
dependencies: flutter: sdk: flutter 100ms_sdk: ^1.0.0 |
Run `flutter pub get` to fetch the dependencies.
Step 3: Initialize 100 MS SDK
In your main Dart file (usually `lib/main.dart`), import the necessary packages and initialize the 100 MS SDK with your API key:
import 'package:flutter/material.dart'; import 'package:hms_sdk/hms_sdk.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { // Initialize 100 MS SDK with your API key HMS.initialize(apiKey: 'YOUR_API_KEY'); return MaterialApp( title: 'Flutter Live Streaming', home: MyHomePage(), ); } } |
Step 4: Implement Live Streaming UI
Create a new Dart file (e.g., `lib/live_stream_page.dart`) to implement the UI for live streaming:
import 'package:flutter/material.dart'; import 'package:hms_sdk/hms_sdk.dart'; class LiveStreamPage extends StatefulWidget { @override _LiveStreamPageState createState() => _LiveStreamPageState(); } class _LiveStreamPageState extends State<LiveStreamPage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Live Streaming'), ), body: Center( child: ElevatedButton( onPressed: () { // Implement logic to start live streaming startLiveStreaming(); }, child: Text('Start Live Streaming'), ), ), ); } void startLiveStreaming() { // Add code to start live streaming using the 100 MS SDK // For example: HMS.startLiveStreaming(); } } |
Step 5: Navigate to the Live Stream Page
Update the `MyHomePage` widget in your main Dart file to navigate to the `LiveStreamPage`:
class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Flutter Live Streaming'), ), body: Center( child: ElevatedButton( onPressed: () { // Navigate to the LiveStreamPage Navigator.push( context, MaterialPageRoute(builder: (context) => LiveStreamPage()), ); }, child: Text('Go to Live Streaming'), ), ), ); } } |
Conclusion
Now, when you run your Flutter app, you should be able to navigate to the Live Streaming page and initiate live streaming using the 100 MS SDK.
Remember to consult the 100 MS SDK documentation for more advanced features and customization options. Additionally, make sure to handle error scenarios and manage the streaming lifecycle appropriately in a production environment.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.