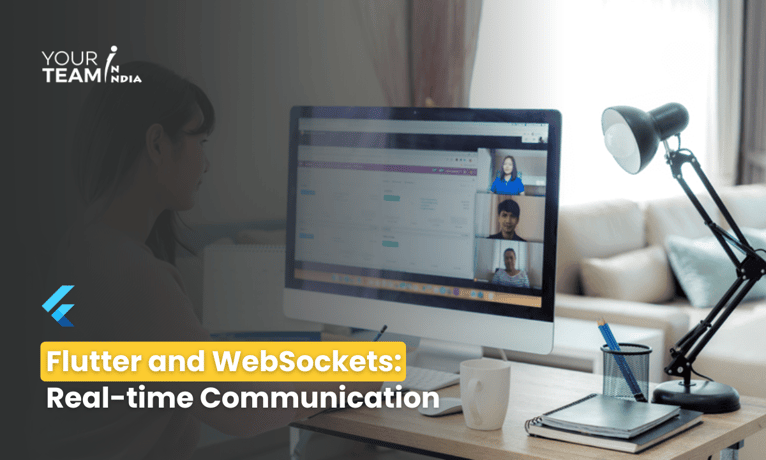
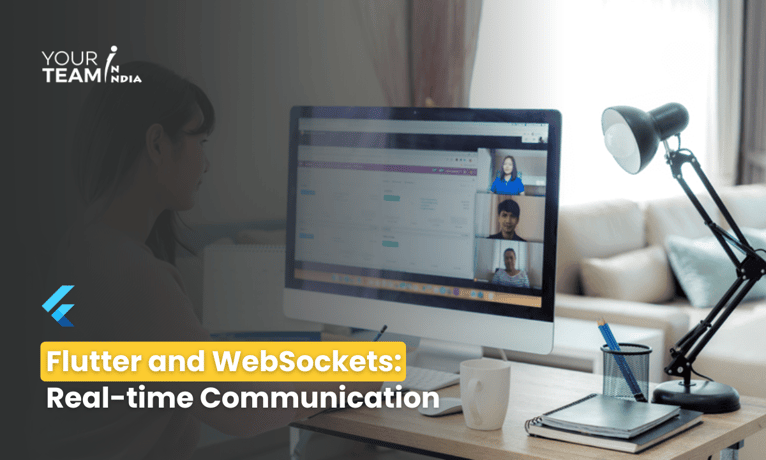
Quick Summary: This comprehensive guide dives into real-time communication in Flutter with WebSockets. It explores how to implement WebSocket connections in Flutter apps, enabling seamless and efficient real-time data exchange for enhanced user experiences.
import 'package:web_socket_channel/web_socket_channel.dart'; |
WebSocket Communication Lifecycle
channel.stream.listen( |
void sendMessage(String message) { |
Real-Time Updates in Widgets
// UI widget that updates in real-time |
final secureChannel = WebSocketChannel.secure( |
Authentication and Authorization
// Send authentication token during WebSocket connection |
Hire Flutter developers to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.
(500+ Clients over 1000+ Projects)
Partner with YTII and build a team within 48 hours