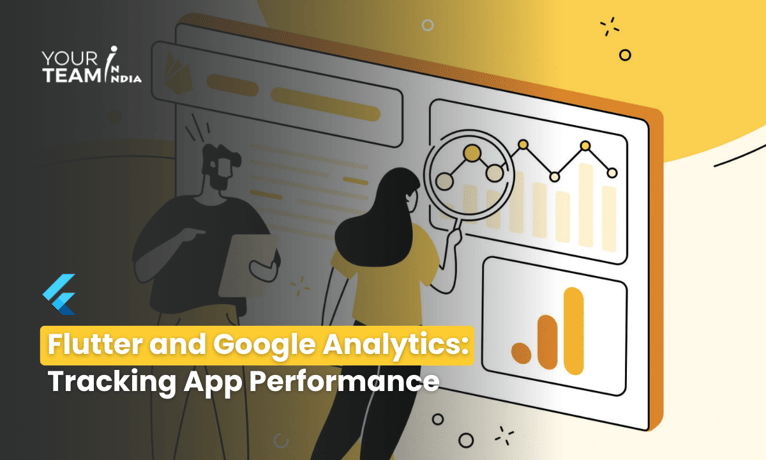
Quick Summary: Unveil the synergy between Flutter and Google Analytics to master tracking app performance. This article illuminates how to integrate Google Analytics into your Flutter app, providing insights into user behavior and app efficiency, which is essential for refining and elevating the overall user experience.
Introduction
- Introduction to the significance of analytics in mobile app development.
- Overview of Google Analytics as a powerful tool for tracking user interactions and app performance.
- The importance of integrating analytics to make data-driven decisions.
Setting Up Google Analytics in a Flutter Project
- Create a Google Analytics Account
- Guidance on creating a Google Analytics account if not already established.
- Navigating to the Google Analytics platform.
- Create a New Property
- Steps to create a new property for your Flutter app within the Google Analytics account.
- Configuring settings for the new property.
- Obtain Tracking ID
- Retrieving the tracking ID associated with the newly created property.
- Where to find the tracking ID within the Google Analytics dashboard.
Integrating Google Analytics with Flutter
- Add the Analytics Package to Your Project
- Overview of the google_analytics package for Flutter.
- Adding the package to the pubspec.yaml file.
# pubspec.yaml |
// Importing Google Analytics package |
Initialize Google Analytics
- Initializing Google Analytics in the Flutter app.
- Configuring the tracking ID during initialization.
// Example: Initializing Google Analytics |
Tracking App Events
- Logging Screen Views
- Tracking screen views to understand user navigation patterns.
- Implementing screen view tracking in Flutter.
// Example: Logging screen views |
Logging Custom Events
- Tracking custom events to monitor specific user interactions.
- Implementing custom event tracking in Flutter.
// Example: Logging custom events |
User Engagement Metrics
- Tracking User Engagement
- Monitoring user engagement metrics such as session duration.
- Implementing code to measure user engagement.
// Example: Tracking user engagement |
Monitoring User Demographics
- Utilizing Google Analytics to gather insights into user demographics.
- Configuring user demographics tracking.
// Example: Configuring user demographics tracking |
Analyzing App Performance
- Monitoring App Crashes
- Using Google Analytics to track and analyze app crashes.
- Setting up crash reporting in Flutter.
// Example: Logging app crashes |
- Analyzing User Journeys
- Leveraging Google Analytics to analyze user journeys within the app.
- Reviewing the Behavior Flow report.
Testing and Debugging
- Testing Analytics Locally
- Configuring a testing environment for Google Analytics in Flutter.
- Avoiding analytics interference during development.
// Example: Setting up testing environment |
Debugging Analytics Issues
- Techniques for debugging common issues with Google Analytics integration.
- Utilizing Google Analytics debugging tools for inspection.
flutter run --enable-software-rendering |
Conclusion
- Recap of key steps in integrating Google Analytics with Flutter for app performance tracking.
- Encouragement for developers to utilize analytics to make informed decisions.
- Reminders about the importance of testing and debugging in analytics integration.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.