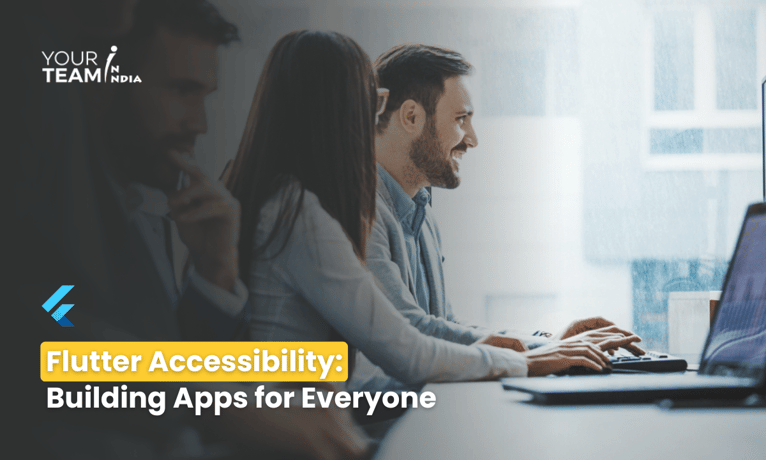
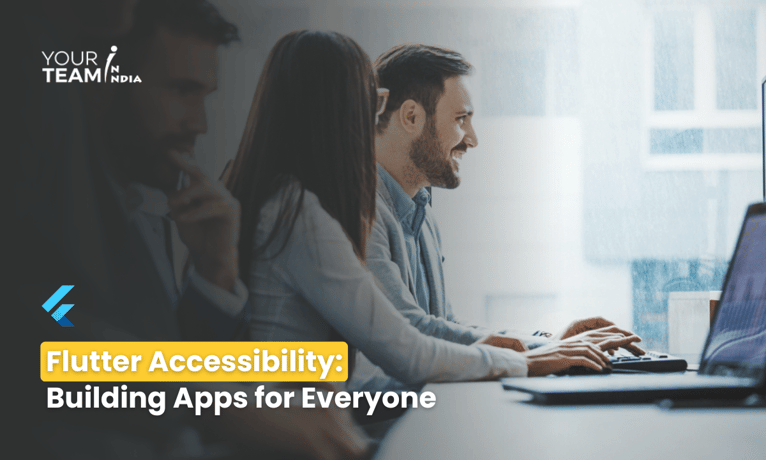
Quick Summary: This comprehensive guide dives into the world of Flutter accessibility. It explores how to build inclusive apps for all users, incorporating accessibility features and best practices to ensure usability and enhance user experience.
AccessibilityTree and Semantics
Semantics( |
Screen Reader Compatibility
// Ensure widgets have meaningful semantics properties |
Container( |
Touchable Areas and Interactive Elements
GestureDetector( |
flutter analyze --preamble |
Hire Flutter developers to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.
(500+ Clients over 1000+ Projects)
Start your 7-days Risk-free trial.