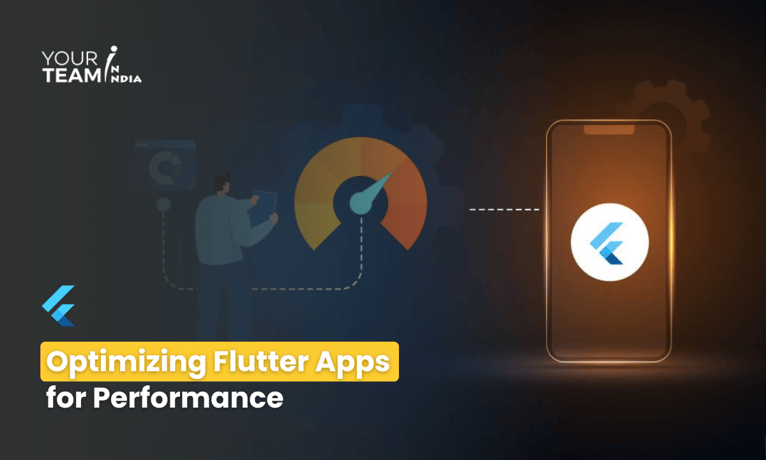
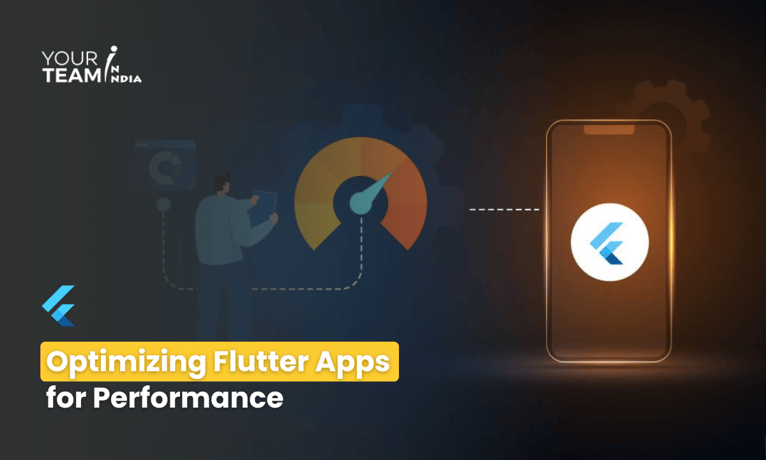
Quick Summary: Dive into the realm of performance optimization in Flutter apps with this comprehensive guide. Explore strategies, techniques, and best practices to enhance app performance, improve user experience, and ensure seamless operation on various devices and platforms.
flutter pub get |
Reducing Widget Rebuilds
class MyWidget extends StatelessWidget { |
Efficient Data Loading
Future<List<Item>> fetchData() async { |
Image.asset('assets/compressed_image.jpg'); |
Minimizing Network Requests
Future<void> fetchData() async { |
Recap of key strategies for optimizing Flutter app performance.
Emphasis on the importance of ongoing performance monitoring and improvement.
Encouragement to implement these optimizations based on the specific needs of the app.
Hire Flutter developers to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.
(500+ Clients over 1000+ Projects)
Partner with YTII and build a team within 48 hours