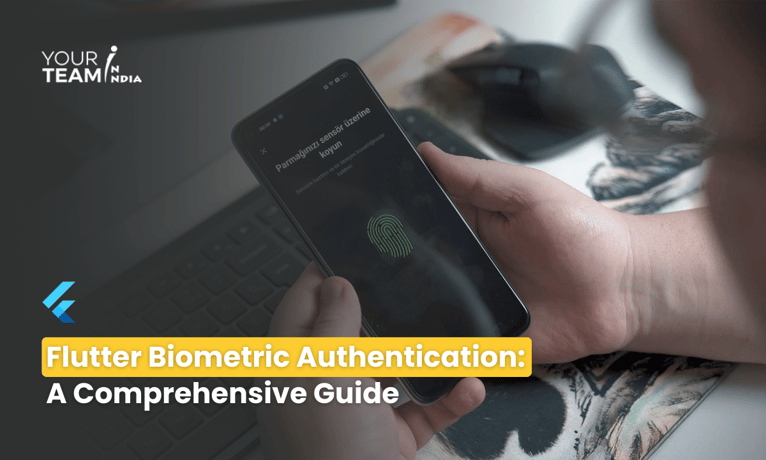
Quick Summary: Delve into the world of Flutter Biometric Authentication with this comprehensive guide. Learn how to implement cutting-edge biometric technologies, such as fingerprint and facial recognition, in Flutter apps, enhancing security and providing a seamless, user-friendly authentication experience for your app users.
Introduction
Biometric authentication has become a popular and secure way to verify the identity of users in mobile applications. Flutter, Google's UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase, provides excellent support for implementing biometric authentication. In this article, we will explore how to integrate biometric authentication into a Flutter app using the `local_auth` package.
Setting Up the Project
Before we dive into the implementation, make sure you have Flutter installed on your machine. Create a new Flutter project using the following command:
flutter create biometric_auth_demo cd biometric_auth_demo |
Now, open the `pubspec.yaml` file and add the `local_auth` package:
dependencies: flutter: sdk: flutter local_auth: ^2.0.1 |
Run `flutter pub get` to fetch and install the dependencies.
Implementing Biometric Authentication
1. Import the necessary packages: Open the `main.dart` file and import the required packages:
import 'package:flutter/material.dart'; import 'package:local_auth/local_auth.dart'; |
2. Create a `LocalAuthentication` instance: Create an instance of `LocalAuthentication` to interact with the biometric authentication services:
final LocalAuthentication _localAuthentication = LocalAuthentication(); |
3. Check for Biometric Support: Before attempting to authenticate, check if the device supports biometric authentication:
Future<void> checkBiometric() async { bool canCheckBiometrics = await _localAuthentication.canCheckBiometrics; print('Biometrics supported: $canCheckBiometrics'); } |
4. Retrieve Available Biometrics: You can also retrieve the types of biometrics available on the device:
Future<void> getAvailableBiometrics() async { List<BiometricType> availableBiometrics = await _localAuthentication.getAvailableBiometrics(); print('Available biometrics: $availableBiometrics'); } |
5. Authenticate with Biometrics: Now, let's implement the biometric authentication:
Future<void> authenticate() async { bool isAuthenticated = false; try { isAuthenticated = await _localAuthentication.authenticate( localizedReason: 'Authenticate to access the app', biometricOnly: true, useErrorDialogs: true, stickyAuth: true, ); } catch (e) { print('Error during biometric authentication: $e'); } if (isAuthenticated) { print('Biometric authentication successful'); } else { print('Biometric authentication failed'); } } |
6. UI Integration: Create a simple UI to trigger biometric authentication:
void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Biometric Auth Demo'), ), body: Center( child: ElevatedButton( onPressed: () async { await checkBiometric(); await getAvailableBiometrics(); await authenticate(); }, child: Text('Authenticate with Biometrics'), ), ), ), ); } } |
Conclusion
We explored how to integrate biometric authentication into a Flutter app using the `local_auth` package. By following the steps outlined above, you can enhance the security of your Flutter applications while providing a seamless and user-friendly authentication experience. Remember to handle errors appropriately and customize the authentication flow to meet the specific requirements of your app.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.