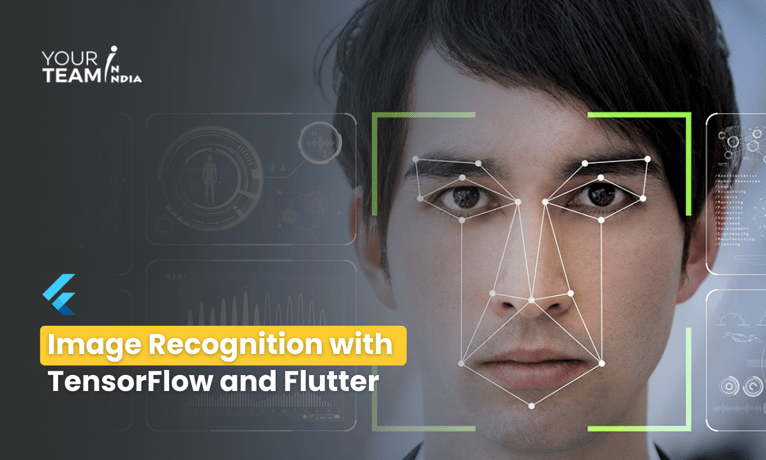
Quick Summary: Explore the fusion of TensorFlow and Flutter for advanced image recognition capabilities in this comprehensive guide. Learn to harness the power of machine learning and mobile app development to create sophisticated, AI-driven applications that can analyze and interpret images with remarkable accuracy and efficiency.
Introduction
Image recognition has become a crucial aspect of many applications, from identifying objects in photos to enabling augmented reality experiences. TensorFlow, an open-source machine learning library, provides a powerful platform for building and deploying machine learning models, including those for image recognition. When combined with Flutter, a popular UI toolkit for building natively compiled applications, you can create seamless and efficient image recognition applications. In this guide, we will walk through the process of building an image recognition app using TensorFlow and Flutter, complete with code snippets.
Setting Up Your Environment
Before diving into the code, you'll need to set up your development environment. Make sure you have the following installed:
- Flutter SDK: Install Flutter by following the instructions on the official Flutter website Flutter Installation Guide (https://flutter.dev/docs/get-started/install)
- Android Studio or Visual Studio Code: Choose your preferred IDE for Flutter development.
- TensorFlow Lite: TensorFlow Lite is a lightweight and efficient version of TensorFlow specifically designed for mobile and edge devices. Follow the instructions to integrate TensorFlow Lite into your Flutter project TensorFlow Lite Flutter Plugin (https://pub.dev/packages/tflite)
Building the Flutter App
Now that your environment is set up, let's create a new Flutter app. Open your terminal and run the following commands:
flutter create image_recognition_app cd image_recognition_app |
Next, open the `pubspec.yaml` file and add the TensorFlow Lite package:
dependencies: flutter: sdk: flutter tflite: ^1.0.0 |
Save the file and run:
flutter pub get |
This will fetch the TensorFlow Lite package and make it available for your Flutter project.
Integrating TensorFlow Lite for Image Recognition
In your Flutter app, create a new Dart file (e.g., `image_recognition_screen.dart`) and add the following code:
import 'package:flutter/material.dart'; import 'package:tflite/tflite.dart'; class ImageRecognitionScreen extends StatefulWidget { @override _ImageRecognitionScreenState createState() => _ImageRecognitionScreenState(); } class _ImageRecognitionScreenState extends State<ImageRecognitionScreen> { List<dynamic>? _recognitions; @override void initState() { super.initState(); loadModel(); } Future<void> loadModel() async { await Tflite.loadModel( model: 'assets/model.tflite', // Replace with the path to your TensorFlow Lite model labels: 'assets/labels.txt', // Replace with the path to your labels file ); } Future<void> classifyImage(String imagePath) async { final List<dynamic> recognitions = await Tflite.runModelOnImage( path: imagePath, numResults: 5, threshold: 0.5, imageMean: 127.5, imageStd: 127.5, ); setState(() { _recognitions = recognitions; }); } @override void dispose() { Tflite.close(); super.dispose(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Image Recognition'), ), body: Column( children: [ // Add your image preview widget here ElevatedButton( onPressed: () { // Call a function to open the image picker and get the selected image path // For simplicity, you can use the image_picker package // https://pub.dev/packages/image_picker // After obtaining the image path, call classifyImage function // classifyImage(selectedImagePath); }, child: Text('Select Image'), ), if (_recognitions != null) // Display the recognition results in a ListView or any other suitable widget // You can customize the UI based on your app's requirements ListView.builder( shrinkWrap: true, itemCount: _recognitions!.length, itemBuilder: (context, index) { return ListTile( title: Text(_recognitions![index]['label']), subtitle: Text('Confidence: ${(_recognitions![index]['confidence'] * 100).toStringAsFixed(2)}%'), ); }, ), ], ), ); } } |
This code sets up a basic Flutter screen with an "Select Image" button and a section to display image recognition results. The `loadModel` function initializes the TensorFlow Lite model, and the `classifyImage` function processes the selected image and retrieves recognition results.
Obtaining a TensorFlow Lite Model
To run this example, you'll need a pre-trained TensorFlow Lite model for image recognition. You can find models on TensorFlow Hub (https://tfhub.dev/) or train your own using TensorFlow and convert it to TensorFlow Lite. For simplicity, we'll assume you already have a TensorFlow Lite model (`model.tflite`) and a labels file (`labels.txt`).
Running the App
To run your app, connect a device or open an emulator, and run the following command in your terminal:
flutter create my_openai_flutter_app cd my_openai_flutter_app |
This will launch your Flutter app, and you can interact with the image recognition screen.
Remember to handle image selection and implement the necessary UI components based on your app's design. You may also need to tweak the model loading and image classification parameters based on the requirements of your specific TensorFlow Lite model.
Congratulations! You've just built a basic image recognition app using TensorFlow and Flutter. Feel free to enhance and customize the app further to suit your specific use case.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.