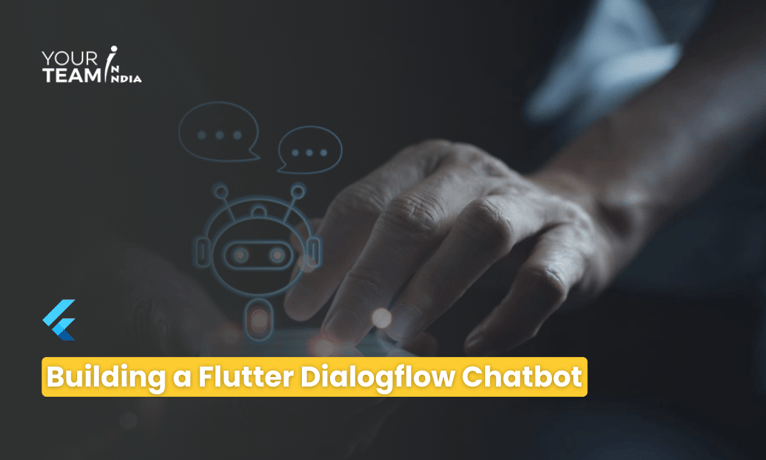
Quick Summary: Embark on a journey to create an interactive Flutter-based chatbot integrated with Dialogflow in this step-by-step guide. Learn to harness the power of Flutter for UI design and Dialogflow's AI capabilities, crafting a responsive and intelligent chatbot for a seamless user engagement experience.
Introduction
In the ever-evolving landscape of mobile app development, creating interactive and engaging chatbots has become a popular trend. Flutter, Google's UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase, provides a powerful platform for developing such chatbots. In this article, we'll explore how to integrate a Flutter app with Dialogflow, a natural language understanding platform, to build a chatbot. We'll walk through the process step by step, including relevant code snippets.
Prerequisites
Before diving into the implementation, make sure you have the following prerequisites:
- Flutter Installed: Make sure you have Flutter installed on your development machine. You can follow the instructions on the official Flutter website (https://flutter.dev/docs/get-started/install).
- Dialogflow Account: Create an account on Dialogflow (https://dialogflow.cloud.google.com/) and set up a new agent.
- Dialogflow API Key: Obtain a Dialogflow API key to authenticate your requests. Follow the instructions in the Dialogflow documentation to get your API key.
Setting up the Flutter Project
- Create a new Flutter project:
flutter create my_dialogflow_chatbot |
- Add the necessary dependencies in your `pubspec.yaml` file:
dependencies: flutter: sdk: flutter http: ^0.13.3 googleapis: ^4.0.0 googleapis_auth: ^2.0.0 |
- Run `flutter packages get` to install the dependencies.
Implementing the Chat Screen
Create a new Dart file for the chat screen, e.g., `chat_screen.dart`. This screen will display the chat interface.
import 'package:flutter/material.dart'; class ChatScreen extends StatefulWidget { @override _ChatScreenState createState() => _ChatScreenState(); } class _ChatScreenState extends State<ChatScreen> { TextEditingController _messageController = TextEditingController(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Dialogflow Chatbot'), ), body: Column( children: [ // Display Chat Messages Expanded( child: ListView.builder( // Implement logic to display chat messages ), ), // Input Field for Sending Messages Padding( padding: const EdgeInsets.all(8.0), child: Row( children: [ Expanded( child: TextField( controller: _messageController, decoration: InputDecoration( hintText: 'Type a message...', ), ), ), IconButton( icon: Icon(Icons.send), onPressed: () { // Implement logic to send messages to Dialogflow }, ), ], ), ), ], ), ); } } |
Integrating Dialogflow API
- Install the `http` package to make HTTP requests:
flutter pub add http |
- Import the necessary packages in `chat_screen.dart`:
import 'package:http/http.dart' as http; import 'dart:convert'; |
- Create a function to send messages to Dialogflow:
Future<void> sendMessageToDialogflow(String message) async { final response = await http.post( Uri.parse( 'https://dialogflow.googleapis.com/v2/projects/YOUR_PROJECT_ID/agent/sessions/YOUR_SESSION_ID:detectIntent', ), headers: { 'Content-Type': 'application/json', }, body: jsonEncode({ 'queryInput': { 'text': { 'text': message, 'languageCode': 'en', }, }, }), ); if (response.statusCode == 200) { // Handle the response from Dialogflow print('Dialogflow Response: ${response.body}'); } else { // Handle errors print('Error: ${response.reasonPhrase}'); } } |
- Call this function when sending a message:
IconButton( icon: Icon(Icons.send), onPressed: () { sendMessageToDialogflow(_messageController.text); // Implement logic to update chat messages _messageController.clear(); }, ), |
Replace `YOUR_PROJECT_ID` and `YOUR_SESSION_ID` with your Dialogflow project ID and a session ID.
Testing the Chatbot
Run your Flutter app and navigate to the `ChatScreen`. Enter a message, press send, and observe the Dialogflow response in the console.
Congratulations! You've successfully integrated a Flutter app with Dialogflow to build a chatbot. Feel free to enhance the chat interface and customize the Dialogflow integration based on your specific use case.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.