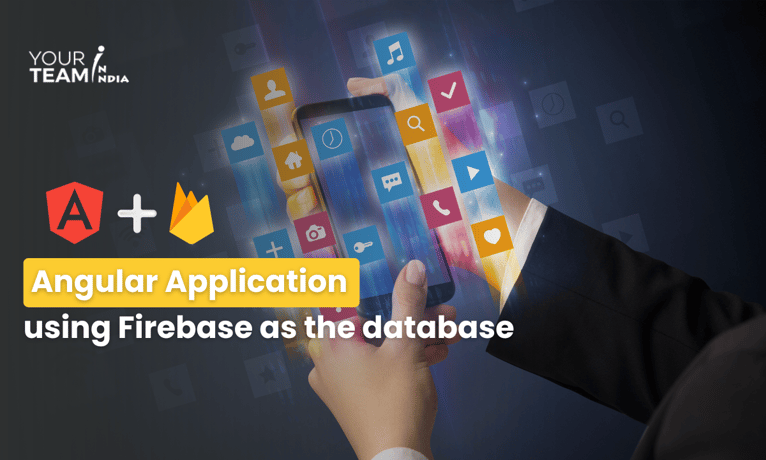
This blog post talks about the perfect partnership between Angular and Firebase. You'll learn how to create a dynamic, real-time web application using Angular as your front-end framework and Firebase as your robust, serverless database. Whether you're a seasoned developer or just getting started, this guide will equip you with the knowledge to build responsive, scalable, and data-driven applications effortlessly.
Key Takeaways
- Discover how Angular and Firebase can seamlessly work together to create dynamic web applications.
- Understand how Firebase's serverless architecture ensures your app can grow without infrastructure worries.
- Explore Firebase's built-in user authentication and security features to protect your application and data.
What Is a Firebase?
Firebase is a Backend-as-a-Service (BaaS) app development platform that provides hosted backend services such as real-time database, cloud storage, authentication, crash reporting, analytics, and so on. It is built on Google's infrastructure and scales automatically.
There are two different ways you can use Firebase in your projects:
- Using Firebase JS SDK
- Using React Native Firebase
React Native supports both the JS SDK and the native SDK. The following sections will guide you through when to use which SDK and all the configuration steps required to use Firebase in your Expo projects.
INITIAL SETUP:
1. First, we need node.js. Install it from here if it is not already installed.
2. Install angular cli
npm install -g @angular/cli
3. Go to firebase
4. Sign Up or Log in there
5. From the top right corner, click Go To Console
6. Click Add Project and create a new project with the desired name.
7. From the project overview page, create a new web app.
8. On the bottom of the General tab, keep the config data for future use.
9. Go to the Cloud Messaging tab and Keep the Server Key and Sender ID.
10. On the bottom of the Web Push Certification section, click Generate key pair and keep the new generated key.
Methods Used to Send Messages to Firebase:
- addDoc()- In order to use the addDoc() method, we need to import three methods from the Firebase Firestore import statement.
- getDatabase() → Firestore database where we want to add data as a document.
- collection() → Where we want to add collection name and database references.
- addDoc() → Where we actually pass our data along with the collection name and db references.
import { getFirestore, collection, addDoc } from "firebase/firestore";
2. updateDoc()- In order to update an existing document using the updateDoc() method, we need three methods:
- getDatabase() → where we want to update a document.
- doc() → where we’ll be passing references of the database, collection and ID of a document that we want to update one of its fields.
- updateDoc() → where we actually pass new data that we want to replace along with the doc() method.
import { getFirestore, doc, updateDoc } from "firebase/firestore";
- getFirestore()
- doc()
- deleteDoc()
import { getFirestore, doc, deleteDoc } from "firebase/firestore";
Adding Firebase to the project:
- The Firebase team offers the package @angular/fire, which provides integration between the two technologies.
ng add @angular/fire
This command installs the @angular/fire package and asks you a few questions. In your terminal, you should see something like:
2. Next, we need to create a Firestore database! Under "Cloud Firestore" click "Create Database."
3. After that, create a database in test mode:
4. Finally, select a region:
5. When you create a new task in the user interface and open Firestore, you should see something like this:
How Firestore is used in a Project:
- When we send a text in a chat, we will text in a list box of a chat, if we share an image, we will see a Photo with a camera icon in a list box and if we share a file (doc, csv, pdf, xlsx, docx, pptx) we will able to see that filename in a list box of that sending and receiving user.
<div class="prof-content align-self-center">
<p class="fs-12 Desc mb-0 text-light angular-with-newlines">
<span *ngIf="!message?.imageUrl"
[innerHTML]="message?.text | detectUrls"></span>
<img class="chat-img cursor-pointer"
[title]="message?.fileName"
(click)="open(0, message?.imageUrl)"
*ngIf="message?.messageType === 'Image'"
[src]="message?.imageUrl" />
<img class="chat-doc cursor-pointer"
[title]="message?.fileName"
(click)="openDocument(message?.imageUrl)"
*ngIf="message?.messageType === 'doc'"
src="/assets/images/doc.svg" />
<img class="chat-doc cursor-pointer"
[title]="message?.fileName"
(click)="openDocument(message?.imageUrl)"
*ngIf="message?.messageType === 'csv'"
src="/assets/images/csv.svg" />
<img class="chat-doc cursor-pointer"
[title]="message?.fileName"
(click)="openDocument(message?.imageUrl)"
*ngIf="message?.messageType === 'pdf'"
src="/assets/images/pdf-file.svg" />
<img class="chat-doc cursor-pointer"
[title]="message?.fileName"
(click)="openDocument(message?.imageUrl)"
*ngIf="message?.messageType === 'xlsx'"
src="/assets/images/xlsx.svg" />
<img class="chat-doc cursor-pointer"
[title]="message?.fileName"
(click)="openDocument(message?.imageUrl)"
*ngIf="message?.messageType === 'docx'"
src="/assets/images/docx.svg" />
<img class="chat-doc cursor-pointer"
[title]="message?.fileName"
(click)="openDocument(message?.imageUrl)"
*ngIf="message?.messageType === 'pptx'"
src="/assets/images/pptx-file.svg" />
<img class="chat-doc cursor-pointer"
[title]="message?.fileName"
(click)="openDocument(message?.imageUrl)"
*ngIf="message?.messageType !== 'Text' &&
message?.messageType !== 'Image' &&
message?.messageType !== 'xlsx' &&
message?.messageType !== 'csv'
&& message?.messageType !== 'pdf' &&
message?.messageType !== 'doc'
&& message?.messageType !== 'docx'
&& message?.messageType !== 'pptx'"
src="/assets/images/document.svg" />
</p>
</div>
</div>
- For detecting the URLs , it is done through a pipe
Use ng generate pipe pipe name
detect-urls.pipe.ts
Conclusion
The fusion of these two technologies has unlocked a realm of possibilities, making it easier than ever to create responsive, real-time, and scalable web applications. Whether you're building an e-commerce platform, a social network, or any other app, Angular and Firebase have your back. Keep the user experience at the heart of your development, and you're sure to achieve remarkable results.
Ready to harness the power of Angular and Firebase for your next project? Hire our AngularJs Developers, and let them build efficient, real-time web applications with the perfect blend of Angular and Firebase.