Quick Summary: ES6 Modules provide a modern way of organizing and managing code in Node.js applications. In this article, we will explore how to effectively use ES6 modules in Node.js, including importing and exporting modules and handling circular dependencies.
Node.js is a popular platform for building scalable, high-performance web applications using JavaScript. With the release of ECMAScript 6 (ES6), developers gained access to new features such as classes, arrow functions, and modules, that made it easier to write clean and maintainable code.
One of the most significant changes introduced in ES6 is the introduction of a new module system, which replaces the CommonJS module system used in previous versions of Node.js. ES6 modules provide a modern, efficient way of organizing and managing code in Node.js applications, making it easier to reuse code across multiple files and projects.
In this article, we will explore how to effectively use ES6 modules in Node.js.
Let’s start!
Key Takeaways
- ES6 modules are a modern and efficient way of organizing and managing code in Node.js applications.
- Importing and exporting modules in ES6 is simple and intuitive, making it easy to reuse code across multiple files and projects.
- When working with ES6 modules, it's important to be aware of potential issues such as circular dependencies and the need to use a transpiler in older versions of Node.js.
Node.JS and ES6 Module - A Preferred Combination
Since 2015, Node.js has been embracing JS ES6 along with the new changes added every year graciously. We even know about two prominent keywords- import and export - which have been introduced to handle working across the packages and the files. Both terms are critical in Node.js. However, it is expected to become standard eventually.
Recommended Read: Advantages of NodeJS
About ES6 Module: What and Why Should You Use It with Node.js?
An ES6 module is a file containing JS code, an automatically strict-mode code, even if you don’t write “use strict.” And, you can use import and export in modules.
Some prominent features of the ES6 module
1. Arrow function
In ES6 module functions, you don’t have to use curly brackets or type the function keyword. Instead, they use a new token ‘=>.’
Syntax: (parameters)=>{Statements}
Arrow functions are majorly used in:
- Return number function
- Return Array function
- Return number function with parameter
- Return object function
2. Object manipulation
Extract object values - for instance, if you have to extract values from ES5, you are expected to write at least 3-4 lines of code, but with ES6, it just needs a single line to do so. Hence, a nice and simple syntax to use in Node.js.
Merging Objects - You can easily merge the object using Object.assign(), which takes both the objects as inputs and outputs the merged objects that you wanted. In addition, the spread operator (...) turns to merge objects into a hassle-free chore for Nodejs development.
Define Object - So, if the name of the key and the variable assigned to that key is similar, it is easier for ES6 to identify which one is the key name and the variable. Don’t worry about doing anything there to make it understood.
3. Template Literal (`)
It provides an alternative syntax to define strings called Template Literals. This syntax utilizes backticks rather than regular quotes or double quotes as delimiters. The prominent advantage of this feature is that it can interpolate variables or expressions using ${expression} inside the string.
`${...}`
4. Module Exports and Imports
- ES6 syntax is more readable, and it comes up with a keyword export default. So, when you want to export a module by default and import the entire module in the source file, this module will do that without hassle.
- You can even import and export multiple variables from a single module using ES6.
For example, if you export:
Export const a=1
Export const b=2
Then, you can import it all together, like:
import {a,b} from testModule
Recommended Read: How To Build CMS Using NodeJS
How is ES6 Module Enabled in Node.js?
You can do that by adding:
“Type”: “Module” to the package.json file.
Once enabled, you can use import and export for work with packages and files.
Import - The keyword ‘import’ works with objects and allows destructuring assignment when importing values. As a result, you can create smaller projects by just including specific values—the import keyword functions in connection with the export keyword.
Export - Export also works with objects, and when working with multiple objects, it creates exported objects created with inputs.
Node.js Doesn’t Support ES6 Module Directly: SO HOW TO USE IT?
Yes, Node.js doesn’t support the ES6 module directly, and even if we try to do so, it will throw out the error. So, for example, if you are trying to import the express module by writing (import express from ‘express’), the Node.js will probably show up an error like this:
Node.js comes with experimental support for ES modules, which you enable by making a few changes in the package.json file. For that, you will need to do this:
A) Open the package. json file and write “type”: “module” and this will enable the ES6 modules for you, looking something like this:
//package.json
{
"name": "index",
"version": "1.0.0",
"description": "",
"main": "index.js",
"type": "module",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC"
}
b) Now, create a file index.js, and using the ES6 import, write the program.
c) Run the index.js file as you type node-experimental-modules-index.js in the terminal.
Need Expert Help? You can Hire NodeJS Developers as well!
(An alternative method you can use)
Using the esm module (world’s most advanced ECMAScript module loader to support ECMAScript modules in Node.js), you can do that:
Create file with .mjs extension (no need to add “type” : “module”)
Write the program directly using ES6 import and execute it, as you type
Node-experiential-modules index.mjs in the terminal.
EXECUTION (Using esm module)
npm install esm
Execute the program earlier written in index.js file. For example, you will need to type node-r esm index.js.
(That was one way of doing it, below is another method to execute it)
Create another file server.js that can load esm before the application. Use this code:
//server.js
require = require("esm")(module);
module.exports = require("./index.js");
What’s the difference up there in both the methods?
In server.js, we import the index.js file carrying the entire program with the purpose of execution.
Finally, type node server.js in the terminal for the execution of the program.
What if your Browser Doesn’t Support ES6?
Node.js Developers know that some browsers don’t support the features of ES6. In that case, you will have to use transpilation of codes, where you will be transforming the code in the language with a similar level of abstraction.
JS makes use of the Babel transpiler to convert ES6 code to ES5, but for that, you will have to install the following packages into your node module.
1. @babel/core: Contains the Node API and requires the hook
2. @babel/preset-env: contains a set of plugins to convert ES6 features to equivalent ES5
3. @babel/register: uses Node require() hook system to compile files when they are loaded.
4. Babel-polyfill: includes a custom regenerator runtime and core-js, which imitates the full ES5 environment and is used in the application.
So, if the old browser you are using doesn’t support the modern ES6 syntax, the entire code written in ES6 will be converted into ES5 to accurately support the browsers you are using.
Once that is done, you can create a babel rc file with the extension .babelrc in the root of your application directory. Then, you can set babel bypassing the package at babel present-env to perform the essential transpilation.
Conclusion
ES6 modules provide a modern and efficient way of organizing and managing code in Node.js applications. With its simple syntax for importing and exporting modules, developers can easily reuse code across multiple files and projects, leading to cleaner and more maintainable code.
However, it's important to be aware of potential issues such as circular dependencies and the need to use a transpiler in older versions of Node.js. By following the best practices for using ES6 modules in Node.js, developers can take advantage of the benefits of this modern module system to create scalable and maintainable applications.
With its powerful features and broad support, ES6 modules are quickly becoming the standard for JavaScript development in Node.js and beyond.
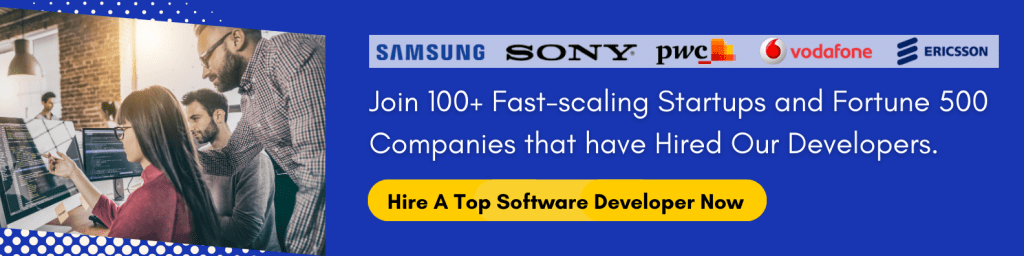
Frequently Asked Questions (FAQs)
What is an ES6 module?
How do I import an ES6 module in Node.js?
import { myFunction } from './myModule.js';
How do I export an ES6 module in Node.js?
export function myFunction() {
// function code here
}
What is a circular dependency, and how do I handle it when using ES6 modules in Node.js?
Can I use ES6 modules in older versions of Node.js?