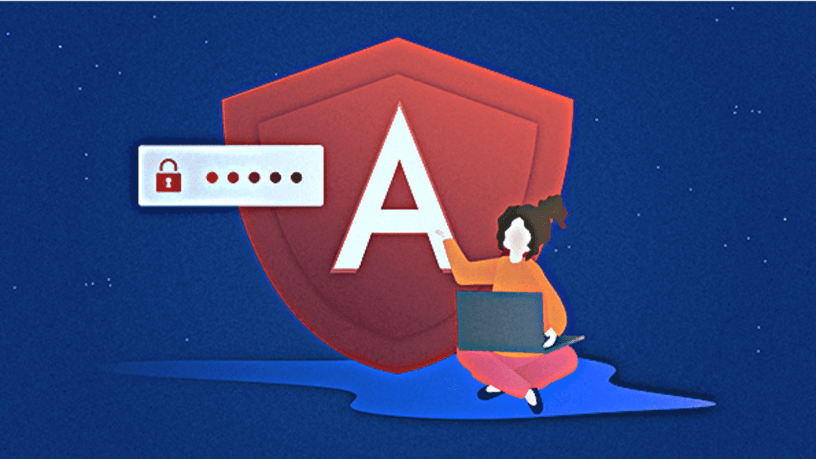
Quick Summary:
Angular has been in the software development world for a good time now. With continuous advancements and innovations, this programming language provides countless ways of developing applications, some better, some worse but definitely faster. This blog will cover the top Angular best practices to help your development process, making it better and more efficient!
Introduction to Angular Best Practices
Angular, developed by Google as a rewrite of AngularJS, is one of the most powerful frameworks for building dynamic programming structures. The basic building blocks of Angular are modules, components, templates, data binding, services, and directives.
Angular has a broad spectrum of internal platforms for designing single-page apps and programming languages such as HTML, CSS, and Typescript. Typescript, the subset of the Javascript framework, is used majorly for developing Angular applications. With the help of Angular programming language, we can create more compatible and robust UI apps.
Due to these functionalities, AngularJS has become one of the most popular backend frameworks in 2023. Also, angular libraries are among the most robust libraries for developers.
This informational blog focuses on the Angular best practices that ensure optimal implementation with the help of Angular programming language for better performance and security.
Ready to elevate your project? Collaborate with expert AngularJS developers for exceptional performance!
Angular Best Practices for Project Setup in 2023
Choosing a good project structure allows for a better angular app development environment. Here are a few angular best practices that would help you ensure the best Angular project setup:
1. Use the Single repository principle
One of the essential best practices in Angular app development is ensuring that no more than one instance of component, directive, or service is created in the same file. Every single file is responsible for a single functionality. Through this, we can ensure that angular code is clean, manageable, and readable.
2. Deploy ES6 Functionality
The complete form of ES6 functionality is ECMAScript 6. This ensures you deal with proper syntax and angular features and implement functions that can help you make your code more modern and clear. In addition, ES6 is continuously modernized with better parts and functionalities and helps prevent memory leaks.
3. Using Angular CLI

Proper utilization of Angular CLI is a practice that can't be ignored. This is one of the most popular tools for developing apps with Angular. Angular CLI is a command-line interface for initializing, developing, maintaining, testing, and debugging Angular apps.
To set up state management in the development environment, angular developers can build an initial-level structure for the overall state management and application state management.
- Ng new to creating an app and is already working out of the box.
- Ng generate-used to generate components, services, and routes.
- Ng serves- used to locate the app locally while developing.
- Ng lint- used to help your angular code shine.
- Ng test- used to run various angular tests on the app.
Recommended Read: Step-by-Step Guide to Hire Angular Developers
Best Angular Component Practices in 2023
The primary purpose of an Angular component is to ensure and encapsulate functionality. If this functionality is repeated several times, you should create smart components as a piece. But you may also want to skip this practice if it's just a code. Here are a few methods for creating components together;
1. Prefixing the component selectors
The Angular CLI builds the component with the app- prefix by default. Customizing component selectors is a good idea, especially in massive projects. It assists you in avoiding conflicts with other imported modules and explicitly identifying the component'scomponent's module (locate the code quickly principle).
Customizing a component prefix could make small reusable components more attractive in tiny apps.
2. Separating component class, CSS, and templates
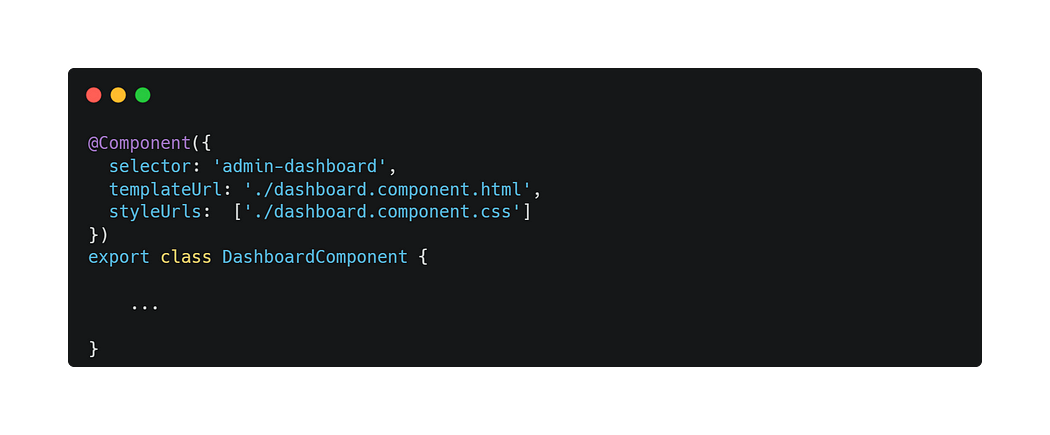
Angular style guide recommends that if the CSS or template is more than three lines, you can move CSS to a style file and HTML in a template. Through the single responsibility principle, you can easily distinguish between components, templates, and services.
3. Using Input and Output with their corresponding decorators
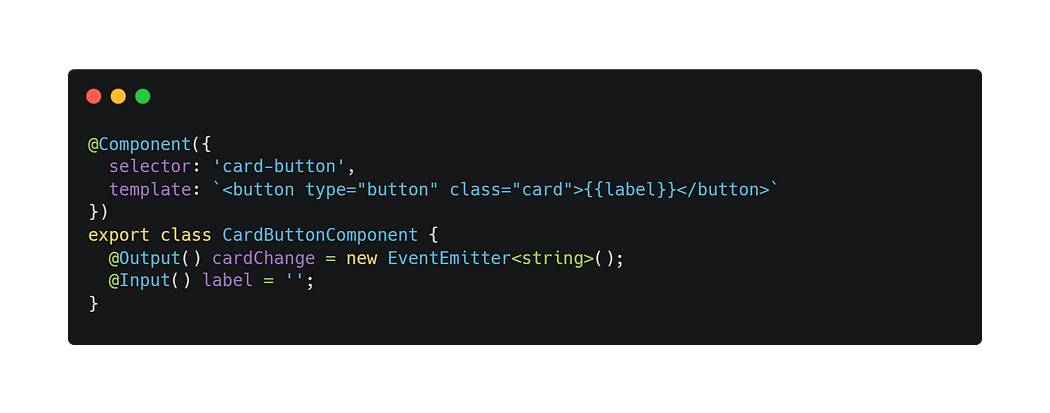
@Input(), @Output() rather than using attributes inside the @Component @Component
4. File Naming
According to the Angular file naming convention, there are two things that you must ensure.
- The name of the files and folders should be set according to their purpose.
- Be consistent with the naming pattern -file features. Filetype.
For instance: consultation.component.ts
- If you want more descriptive tags added to your file, you can use (-) for separating the word the name- book-appointment.component.component.ts
5. Class Naming
While naming the classes, use the upper camel case style with the added suffix representing your file type- TcHomeComponent, AuthService.
6. Folder structure
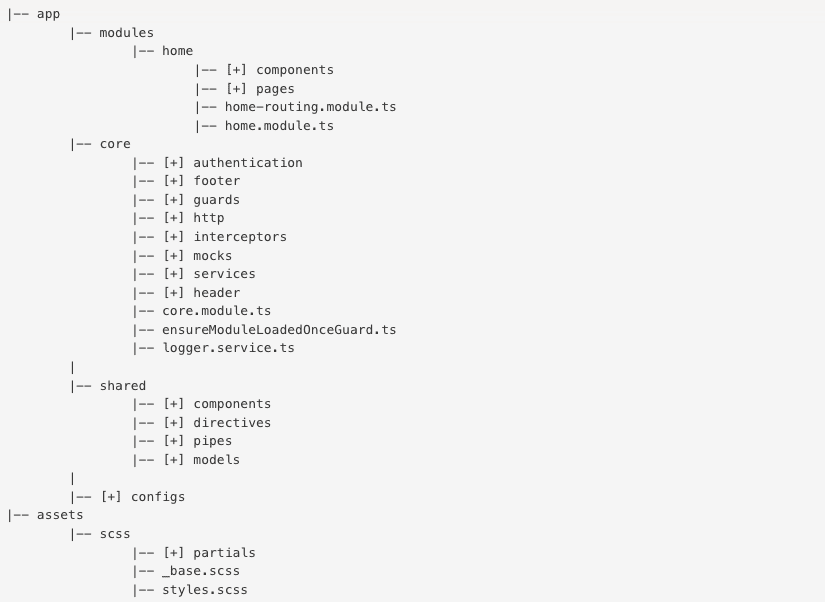
One of the essential things about Angular is deploying modules to group-related features. creating and maintaining a proper folder structure would help you adapt easily to the new changes. Using Angular modules for developing angular structures is one of the best practices.
Each module should consist of a folder and should be named according to its module name. Angular does not distinguish between the different modules and should be classified as our modules for better understanding.
Recommended Read: Difference between Angular and AngularJS
Best Angular Service Practices in 2023
A component should use services for activities that do not require the display or application logic. Services are helpful for activities like retrieving data from a server, verifying user input, and logging directly into the console. Setting up environment variables can help declare individual variables. You may also make your application more versatile by including alternative suppliers of the same type of service as needed in different situations.
Angular does not enforce these standards. Angular, on the other hand, assists you in adhering to these standards by making it simple to component your application logic into services. Dependency injection in Angular makes such services available to components.
Here are three more services angular best practices that you can follow:
1. Service to increase modularity
Here's an example of a service class that logs into the browser console.
src/app/logger.service.ts (class)
content_copy
export class Logger {
log(msg: any) { console.log(msg); }
error(msg: any) { console.error(msg); }
warn(msg: any) { console.warn(msg); }
}
Services can depend easily on other services. For instance, HeroService depends on the Logger service and uses BackendServices to get heroes.
src/app/hero.service.ts (class)
content_copy
export class HeroService {
private heroes: Hero[] = [];
constructor(
private backend: BackendService,
private logger: Logger) { }
getHeroes() {
this.backend.getAll(Hero).then( (heroes: Hero[]) => {
this.logger.log(`Fetched ${heroes.length} heroes.`);
this.heroes.push(...heroes); // fill cache
});
return this. heroes;
} }
2. Dependency injection (DI)
DI is a feature of the Angular framework that allows components to access services and other resources. For example, you can inject a service into a component in Angular to provide that component access to the service.
The @Injectable() decorator in Angular declares a class as a service and allows Angular to inject it as a dependency into a component. The @Injectable() decorator, on the other hand, specifies that an element, class, pipe, or NgModule is dependent on a service.
- The primary mechanism is the injector. Angular provides an application-wide injector and other injectors as needed during the bootstrap phase. You do not need to construct injectors.
- An injector creates dependencies and keeps a container of dependent objects to reuse.
- A provider is a class instructing an injector on how to get or construct a dependency.
3. Registration with the service provider
You must register at least one service provider that is deployed. The provider should be a part of the service's metadata, making that particular service available everywhere, or you can also register providers with specific modules or components.
You should register providers in the metadata of the services ( in the @Injectable() decorator).
- When you provide the service on the root level, Angular creates a single and shared instance of HeroService and injects it into the class that asks for it. This also allows Angular to optimize the app by removing the service from the compiled application.

Best Angular Module Practices in 2023
Modules are essential in an Angular project. Lazy loading and shared model features are two critical parameters for the same. Let's discuss the module angular best practices;
1. Splitting applications into multi-modules
The advice is to divide dumb components of our application into many modules, even though an Angular application would function just fine with a single module. This strategy offers a lot of benefits.
For example, we can employ the lazy-loading functionality, the project structure is more structured, and it is more manageable, readable, and has reusable components.
2. Lazy Loading
It is advised to incorporate a lazy load functionality if our application consists of several modules. The main benefit of a lazy loading strategy is that we load resources as needed rather than all at once. This assists us in speeding up the initial process of backend angular development. [ When a user navigates to one of the lazily loaded modules, it will immediately begin to load.
We'll demonstrate how to configure lazy loading in our module.
const appRoutes: Route[] = [
{ path: ''home'', component: HomeComponent },
{ path: 'owner', loadChildren: "./owner/owner.module#OwnerModule" },
]
In this code example, the HomeComponent loads multiple components eagerly, but the OwnerModule and all the components registered in that module are loading lazily.
3. Utilizing the Shared module file
Registering components, directives, or pipes inside the shared module file is advisable if we use them consistently throughout the project. The shared feature module must then be registered inside the app module. It's crucial to avoid writing services in a shared module that we wish to utilize globally. Such services should be reported either in their feature or app modules.
But if any service is required inside a shared module, the best way is to use the forRoot() method that returns the ModuleWithProviders interface:
Imports and @NgModule and exports part:
export class SharedModule {
static forRoot(): ModuleWithProviders {
return {
ngModule: SharedModule,
providers: [ AnyService ]
};
}
}
Now, we can register this shared module in our app using SharedModule.forRoot().
Best Angular Testing Practices in 2023
A crucial stage in quality assurance is creating high-quality tests for essential functionality. We only advocate developing tests or aimlessly attempting to obtain 100% coverage.
Applications with 100% coverage may still have flaws if the implementation does not account for all edge situations or the tests are inadequate.
Testing Angular apps involves a lot of tips and tactics. Some of them will be covered in this section:
1. E2E Testing
Comparing end-to-end testing with Jasmine's unit/integration testing will reveal significant differences. E2E testing is included in as one of the angular best practices for security. A separate E2E testing project that uses Protractor is included with Angular CLI applications. Protractor is just a wrapper for Selenium, allowing us to write tests in JavaScript and add specific utility methods particular to Angular.
Your application will be created when you begin E2E tests, and a web browser will be launched under your control. The tests will then automatically click on various website items without any human involvement (via WebDriver).
This enables us to quickly write angular coding tests of the smoke-testing variety that automatically run through the critical processes of our application.
Some E2E testing frameworks that are worth checking out are:
- Cypress.io
- WebdriverIO
2. Utilizing TestBud
Because it enables you to offer dummy services for testing, dependency injection is particularly effective during testing. You may observe how certain private services operate. You might be tempted to do something like component["myService"] to get around TS or publicize the service. You may use TestBed to stay away from these impure solutions.
To obtain an instance of a dependency that the component being tested injects, use TestBed.inject. I
n the following example, we will use the UserService class as an environment variable to obtain an instance of UserTestingService. This needs to coincide with the pass used to inject the user object into the model in the header component function Object() { [native code] }.
3. Writing unit testing for components, services, and modules
Although creating tests may seem like an extra burden, it is an essential skill for all engineers. Local unit tests and the final automated end-to-end tests are crucial for early issue detection.
Creating tests may need some of your initial efforts, but it will be worthwhile in the long run, for instance, when you need to compare the functionality of the old and new versions after making modifications.
Test writing has the following primary advantages:
- Faster development, fewer problems, and support for test-driven development.
Best Angular Performance Practices in 2023
Angular performance matters for a considerable time. Therefore, while developing apps for backend as well as front end, it is essential to deploy the angular best practices to ensure the best performance:
1. Take care of performance with Angular Differs API calls
Angular Differs API has yet to gain as much popularity as it should have. The primary idea is to have a clear picture of what has changed in angular coding and angular best practices instead of just the information.
Angular differs APIs have the following two different API options:
- IterableDiffer, which records modifications made to the iterable over time and displays ways to respond to these modifications,
- The object is tracked over time by KeyValueDiffers, which also offers ways to respond to these changes.
Performance is the most significant advantage of using the Angular Differs API.
2. Ahead of time to improve load time performance
The angular components are easier to understand and interpret if the Angular HTML and Typescript code is converted into efficient Javascript code. The process is done by compilers such as JIT and AOT; Angular provides that.
3. Building optimize flag
Specify the "build-optimizer" flag whenever "angular-cli if you use angular-cli for your production build. This would create minor code by disabling the chucreator by the vendor. Whenever you have a minor code, the size of the app automatically reduces to minimalist. This helps create better performance, speed, and enhanced user experience.
Best Angular Security Practices in 2023
Security in Angular backend development is more about the built-in capabilities that the framework provides than application-level security.
How can Angular programming for backend development protect your website from security threats? What are the angular security best practices?
Your client-side platform needs security for a variety of reasons, one of which is cross-site scripting. This kind of scripting assault can spread to the client-side controls and alter users' web pages.
On the other side, several built-in safeguards offered by the Angular framework can stop these assaults.
Some of the essential tools used for Security Auditing are:
- SecurityHeaders.io to analyze the HTTP response headers
- BurpSuite for manual security testing
- SonarQube for Code Quality & Code Security
Web security is also important when protecting a website from attacks. Here are three best practices to avoid security vulnerabilities in the Angular best apps.
1. Preventing Cross-Site Scripting
In web applications, to prevent malicious attacks, Cross-Site Scripting(XXS) is used for security vulnerability. This process sends a script to the attacker and the user simultaneously. The primary purpose of the same is to prevent the user from accepting the malicious script.
As the website is trusted, the users accept the website, and malicious scripts are executed.
To avoid cross-site scripting attacks, it is required to prevent untrusted scripts and allow only clean code from injecting the malicious scripts into the DOM tree. Here are a few ways in which you can stop such attacks:
- At server-side processing, you need to clear all the data containing HTML and Javascript tags before lending the HTTP response.
- You would also limit yourself from generating the angular templates using server-side processing. This could result in the insertion of the template.
2. Using route guides on the navigation
We can either accept or reject the URL requests by the users by working on the route guard interface. These are also used as a boolean concept, returning a value that can be used to navigate to the requested URL.
Different types of route guards, CanActivate, CanActivateChild, CanDeactivate, and Resolve
Recommended Read: Angular Authentication Using Route Guards
3. Avoid making changes in the Angular Core Module
Changing and modifying the files in the Angular core module can affect security and lead to protection issues. Furthermore, the modifications made in the default content of the Angular development can harm the existing functionalities and even change the behavior of the current version.
Conclusion
Building web applications and upgrading them is an ongoing process. There's always some scope to improve the way you use a programming language and build apps with the same. This comprehensive blog covers all the Angular best practices for developing efficient Angular backend apps. You can utilize the tips to reap the maximum benefits from the same.
This list of best practices would allow you to get started and apply the same pattern of things to the project to make your application clean and better.
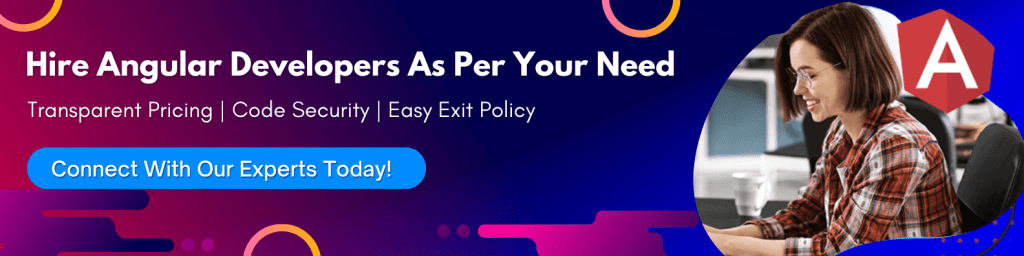
Frequently Asked Questions (FAQs) on Angular Best Practices
What are Angular's best practices?
Why are Angular best practices important?
What are some key Angular best practices?
Can Angular best practices improve the performance of my application?