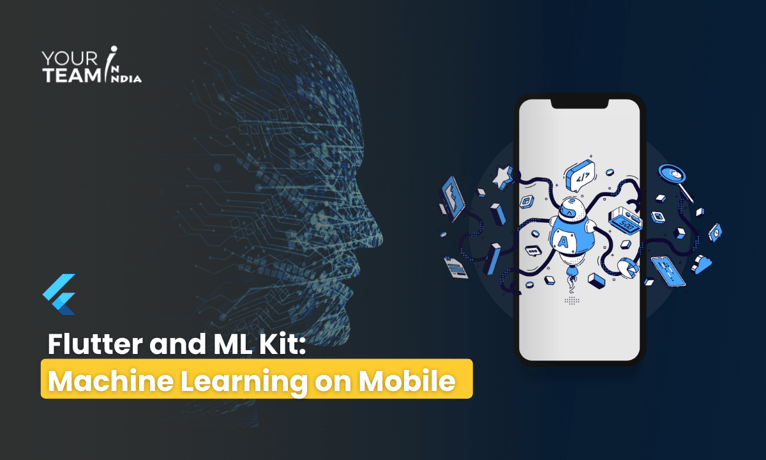
Quick Summary: Venture into the innovative intersection of Flutter and ML Kit, where machine learning meets mobile development. This article guides you through the integration process, enabling Flutter apps to harness the power of ML for enhanced features, from image recognition to predictive analytics.
Introduction
- Introduction to machine learning on mobile devices.
- Overview of ML Kit as a machine learning framework for mobile apps.
- The synergy of Flutter and ML Kit for building intelligent and feature-rich applications.
Understanding ML Kit
- What is ML Kit?
- Explanation of ML Kit as a machine learning SDK by Google for mobile app development.
- Overview of ML Kit's pre-trained models and APIs.
- Supported Features and Use Cases
- List of supported machine learning features and use cases in ML Kit.
- Examples of ML Kit's capabilities, such as image labeling, text recognition, face detection, and more.
Integrating ML Kit with Flutter
- Adding ML Kit Dependency
- Adding the ML Kit plugin to the Flutter project.
- Configuring the pubspec.yaml file.
# pubspec.yaml |
// Importing ML Kit package |
Initializing ML Kit
- Initializing ML Kit in a Flutter app.
- Configuring necessary settings.
// Example: Initializing ML Kit |
ML Kit Features in Flutter
- Image Labeling
- Implementing image labeling with ML Kit in Flutter.
- Extracting labels from images.
// Example: Image labeling with ML Kit |
Text Recognition
- Implementing text recognition with ML Kit in Flutter.
- Extracting text from images.
// Example: Text recognition with ML Kit |
Face Detection
- Implementing face detection with ML Kit in Flutter.
- Extracting facial features and information.
// Example: Face detection with ML Kit |
Customizing ML Kit Models
- Using Custom Models
- Overview of using custom machine learning models with ML Kit.
- Configuring Flutter apps to work with custom models.
// Example: Using custom models with ML Kit |
Model Personalization
- Personalizing ML Kit models for specific use cases.
- Training and fine-tuning models for improved accuracy.
// Example: Model personalization with ML Kit |
Offline Support with ML Kit
- Using On-Device Models
- Leveraging on-device machine learning models for offline scenarios.
- Configuring ML Kit to use locally stored models.
// Example: Using on-device models with ML Kit |
Caching Model Results
- Implementing caching mechanisms for ML Kit model results.
- Ensuring efficient use of locally processed data.
// Example: Caching ML Kit model results |
Testing and Debugging
- Testing ML Kit Integration Locally
- Configuring a testing environment for ML Kit in Flutter.
- Utilizing sample data for testing ML Kit features.
# Example: Testing ML Kit locally |
Debugging ML Kit Issues
- Techniques for debugging common issues with ML Kit integration.
- Utilizing Flutter's debugging tools for machine learning inspection.
flutter run --enable-software-rendering |
Let's create a simple example demonstrating how to perform text recognition using ML Kit in a Flutter app. This example assumes you have set up a Flutter project and added the firebase_ml_vision dependency.
import 'package:flutter/material.dart'; |
In this example, we initialize ML Kit, create a text recognizer, and use it to process an image for text recognition. The recognized text is then printed to the console. The Flutter app provides a button to trigger the text recognition process.
Make sure to replace 'path/to/your/image.jpg' with the actual path to the image you want to process. You can use an image picker or load an image from your assets, depending on your use case.
Conclusion
- Recap of key steps in integrating ML Kit with Flutter for machine learning on mobile.
- Encouragement for developers to explore various machine learning use cases in Flutter apps.
- Reminders about the importance of testing and optimizing for performance in ML Kit integration.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.