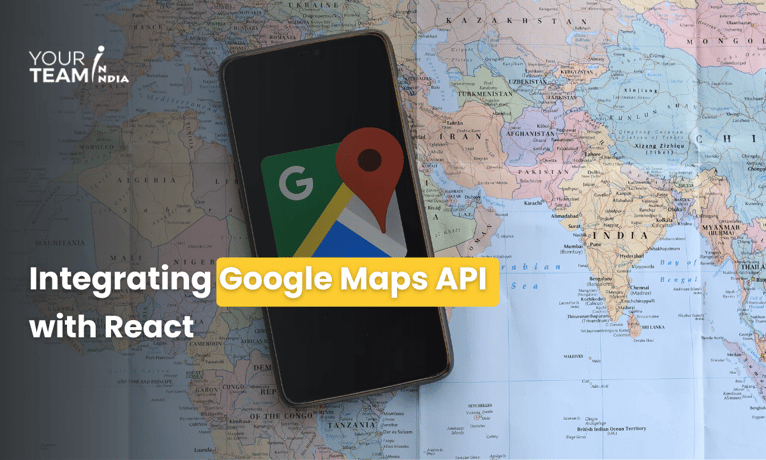
Quick Summary: Discover the power of combining Google Maps API with React in our comprehensive guide. Learn how to seamlessly integrate interactive maps into your web applications, harnessing the full potential of location-based data and user experiences. Explore step-by-step instructions and best practices to elevate your projects with geospatial functionality.
React, a popular JavaScript library for building user interfaces provides an excellent platform for integrating various third-party services, including maps. Google Maps is a widely used mapping service, and integrating it with a React application can enhance its functionality significantly. In this article, we will explore the steps to integrate Google Maps API with a React application.
Prerequisites
Before diving into the integration process, make sure you have the following prerequisites:
- Node.js and npm: Ensure that you have Node.js installed on your system, as it includes npm (Node Package Manager). You can download Node.js from the official website (https://nodejs.org/).
- Create React App: You should have a React application set up. If you don't already have one, you can create a new React app using Create React App. Install it globally using the following command:
npm install -g create-react-app
npx create-react-app google-maps-react-app
- Replace google-maps-react-app with your preferred app name.
- Google Maps API Key: You'll need a Google Maps API key to access Google Maps services. To obtain an API key, follow these steps:
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Enable the "Google Maps JavaScript API" for your project.
- Create an API key and restrict it if needed (for security)
Setting up Google Maps API in React
Now that you have your React app and API key ready let's integrate Google Maps into your application.
1. Install React Google Maps Library
To make integration easier, you can use the Google Maps-react library, which is a wrapper for the Google Maps JavaScript API. Install it using npm or yarn:
npm install google-maps-react
# or
yarn add google-maps-react
2. Create a Google Maps Component
Now, let's create a new component that will render the Google Map. In your React project, navigate to the src folder and create a new component file, such as GoogleMap.js. Here's a basic example of how your component can look:
// src/components/GoogleMap.js
import React from 'react';
import { Map, GoogleApiWrapper } from 'google-maps-react';
class GoogleMap extends React.Component {
render() {
const mapStyles = {
width: '100%',
height: '400px',
};
return (
<Map
google={this.props.google}
zoom={14} // Adjust the initial zoom level
style={mapStyles}
initialCenter= // Set the initial center of the map
>
{/* Add map content here */}
</Map>
);
}
}
export default GoogleApiWrapper({
apiKey: process.env.REACT_APP_GOOGLE_MAPS_API_KEY,
})(GoogleMap);
3. Styling the Map
You can customize the map's appearance by modifying the mapStyles object in the GoogleMap.js component. Adjust the width, height, and other properties to fit your application's design.
4. Adding Markers and Additional Features
To make your map more useful, you can add markers, shapes, or other features. Refer to the Google Maps JavaScript API documentation for guidance on how to implement these features.
5. Running Your Application
To see your React application with the integrated Google Map, run the following command from your project's root directory:
npm start
# or
yarn start
Conclusion
Integrating Google Maps into a React application provides powerful location-based functionality to enhance user experiences. By following the steps outlined in this guide, you can easily set up Google Maps in your React project and begin building location-aware applications. Remember to explore the Google Maps JavaScript API documentation for more advanced features and customization options.
Explore the proficiency and hire our ReactJs developers to create applications that offer potent and user-centric solutions.