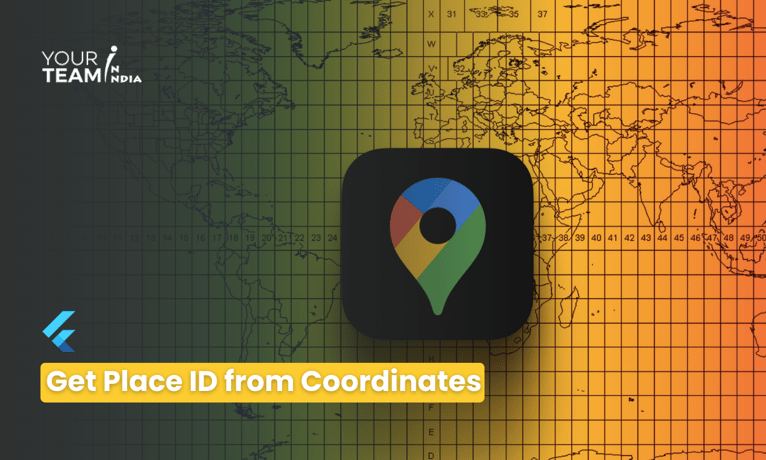
Quick Summary: Obtaining a Place ID from coordinates simplifies location-based tasks. By leveraging various mapping APIs like Google Maps, one can efficiently convert latitude and longitude values into unique identifiers, facilitating precise location referencing for diverse applications.
Introduction
In today's digital age, where location-based services are ubiquitous, retrieving a Place ID from geographic coordinates has become a fundamental aspect of many applications. Whether for mapping services, geotagging, or location-based analytics, the ability to convert latitude and longitude coordinates into unique identifiers streamlines processes and enhances user experiences.
This article explores the techniques and tools available for obtaining Place IDs from coordinates, focusing on the practical applications and benefits. From leveraging the robust capabilities of mapping APIs such as Google Maps to understanding the underlying mechanisms of location-based data retrieval, this guide offers insights to navigate the complexities of location-based programming effortlessly.
To obtain a Place ID from Google's Places API using latitude and longitude coordinates, you can follow these steps:
- Set Up Your Google Cloud Project:
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Enable the "Places API" for your project. You can do this by navigating to the API Library and searching for "Places API."
- Create API Credentials:
- In the Google Cloud Console, navigate to the "APIs & Services" > "Credentials" section.
- Click on "Create Credentials" and select "API Key." This will generate an API key that you will use to authenticate your requests.
- Use the Places API:
- You can make a request to the Places API to retrieve the Place ID based on latitude and longitude. You can use the "Place Search" or "Place Details" request for this purpose.
Example using the Place Search request (HTTP GET):
To retrieve a Place ID from Google's Places API using Flutter and the Dio library, you can follow these steps. Make sure you have added the dio library to your Flutter project by adding it to your pubspec.yaml file:
dependencies: |
Here's a Flutter code example to fetch a Place ID based on latitude and longitude coordinates using Dio:
import 'package:dio/dio.dart'; |
Make sure to replace 'YOUR_API_KEY' with your actual Google API Key, and customize the latitude, longitude, and radius parameters as needed for your specific use case.
Ensure that you have proper error handling and UI integration as per your app's requirements. This code will make an HTTP GET request using Dio to Google's Place API and extract the Place ID from the response.
Conclusion
In conclusion, the process of obtaining a Place ID from coordinates unlocks a myriad of possibilities for location-based applications. By harnessing the power of mapping APIs and understanding the nuances of geographic data conversion, developers and businesses can seamlessly integrate precise location referencing into their projects. Embracing this technology empowers users and enhances the efficiency and accuracy of location-driven solutions across various domains.
Hire Flutter developers to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.