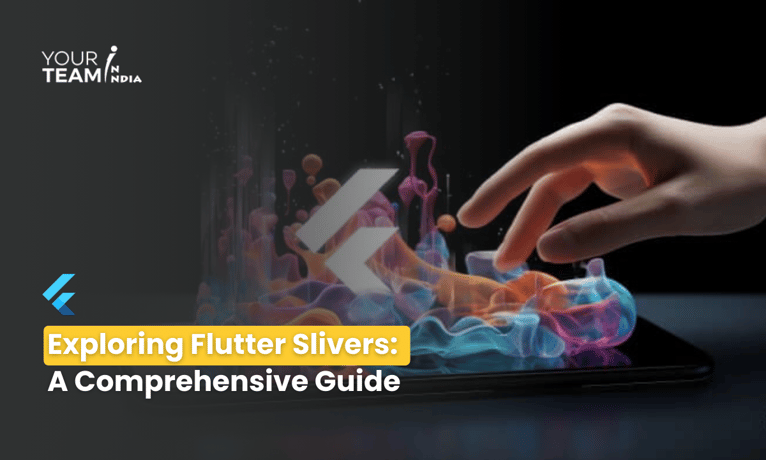
Quick Summary: Dive into the dynamic world of Flutter Slivers with this comprehensive guide. Understand how these flexible and powerful components can transform the way you design scrollable areas in your Flutter applications, offering enhanced user experience and sophisticated layouts for versatile app development.
Flutter, Google's UI toolkit, offers a rich set of widgets to create beautiful and responsive user interfaces. Among these, the Sliver widgets play a crucial role in building flexible and dynamic layouts. In this article, we'll explore Flutter Slivers and provide code snippets to illustrate their usage.
Understanding Slivers
In Flutter, a "Sliver" is a portion of a scrollable area. Slivers are lightweight, flexible, and can be composed to create complex scrollable UIs. They work seamlessly with various scrolling widgets like `CustomScrollView`, `NestedScrollView`, and more.
Basic Anatomy of a Sliver: A Sliver typically consists of three main components:
- SliverAppBar: This is a special type of app bar that integrates with Slivers. It can expand and contract based on the scroll position.
- SliverList: Represents a linear list of items. It is an efficient way to display a large number of children without creating all the widgets at once.
- SliverGrid: Similar to SliverList, it arranges its children in a grid.
Implementing a Basic Sliver
Let's start with a simple example using `CustomScrollView` with a `SliverAppBar` and a `SliverList`.
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: CustomScrollView( slivers: [ SliverAppBar( expandedHeight: 200.0, flexibleSpace: FlexibleSpaceBar( title: Text('Flutter Slivers'), background: Image.network( 'https://example.com/image.jpg', fit: BoxFit.cover, ), ), ), SliverList( delegate: SliverChildBuilderDelegate( (BuildContext context, int index) { return ListTile( title: Text('Item $index'), ); }, childCount: 50, ), ), ], ), ), ); } } |
In this example, we create a `CustomScrollView` with a `SliverAppBar` containing an image and a title. Below the app bar, there's a `SliverList` displaying a list of 50 items using `SliverChildBuilderDelegate`.
Advanced Sliver Techniques
Custom SliverGrid
SliverGrid( gridDelegate: SliverGridDelegateWithFixedCrossAxisCount( crossAxisCount: 2, crossAxisSpacing: 8.0, mainAxisSpacing: 8.0, childAspectRatio: 1.0, ), delegate: SliverChildBuilderDelegate( (BuildContext context, int index) { return Card( child: Center( child: Text('Item $index'), ), ); }, childCount: 20, ), ), |
Here, we use `SliverGrid` to create a grid layout with a custom delegate.
Nested Scroll Views
NestedScrollView( headerSliverBuilder: (BuildContext context, bool innerBoxIsScrolled) { return [ SliverAppBar( expandedHeight: 200.0, pinned: true, flexibleSpace: FlexibleSpaceBar( title: Text('Nested Scroll View'), background: Image.network( 'https://example.com/image.jpg', fit: BoxFit.cover, ), ), ), ]; }, body: SliverList( delegate: SliverChildBuilderDelegate( (BuildContext context, int index) { return ListTile( title: Text('Item $index'), ); }, childCount: 50, ), ), ), |
In this example, we use `NestedScrollView` to create a scroll view with a collapsible app bar.
Conclusion
Flutter Slivers provides a powerful way to build complex and responsive UIs. Whether you're creating a simple list or a sophisticated grid, Slivers offers the flexibility needed for diverse design requirements. Experiment with these code snippets to enhance your Flutter app's scrolling experience.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.