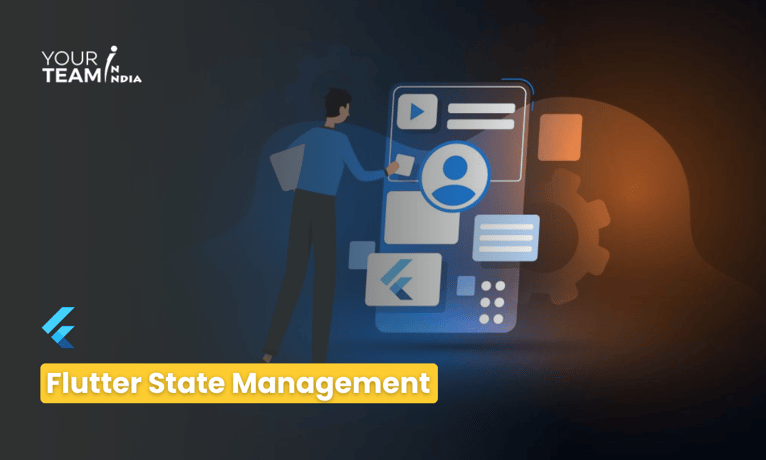
Quick Summary: This comprehensive guide delves into the complexities of Flutter state management. Explore various state management techniques, including setState, Provider, Bloc, and more. Learn how to effectively manage and update the state of your Flutter applications for optimal performance and user experience.
Introduction
- Brief overview of the importance of state management in Flutter apps.
- Explanation of why good state management is crucial for app performance and maintainability.
Flutter State Management Options
1) setState
- Explanation of the basic state management provided by the Flutter framework.
- It is important to use setState to manage local states within widgets.
- Code example demonstrating the use of setState.
class CounterApp extends StatefulWidget { |
2) Provider Package
- Introduction to the Provider package for more scalable state management.
- Advantages of using Provider for managing global and local state.
- Step-by-step guide on integrating Provider into a Flutter app.
- Code example illustrating Provider usage.
// pubspec.yaml |
3) Riverpod
- Introduction to Riverpod as an alternative to Provider.
- Benefits and use cases for Riverpod.
- Implementation guide with code examples.
// pubspec.yaml |
Choosing the Right State Management for Your App
- Factors to consider when selecting a state management approach.
- Comparison of different state management options based on app complexity and requirements.
Conclusion
- Recap of state management options in Flutter.
- Advice on when to use each approach based on the app's requirements.
Hire Flutter developers to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.