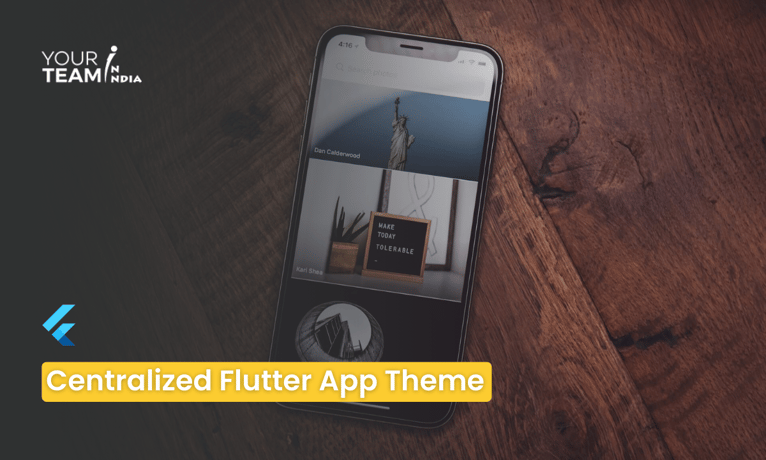
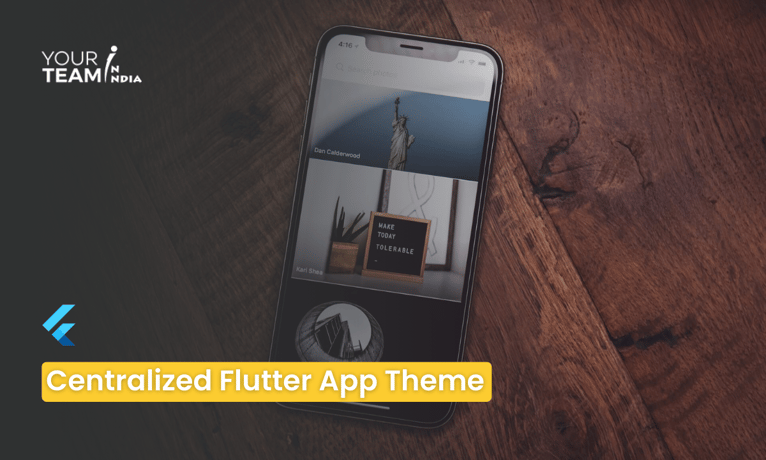
Quick Summary: Dive into the concept of centralized Flutter app themes with this insightful article. Explore how to create and manage a unified theme for your Flutter application, streamlining design consistency and allowing for easy theme customization across the entire app.
In Flutter, creating a centralized and well-structured theme for your app is a good practice. This makes it easier to maintain consistency across your app's design and allows for quick and systematic changes.
Here are some best practices for creating a centralized theme in Flutter:
ThemeData myTheme = ThemeData( |
2. Create a Theme Widget: Wrap your entire app with a Theme widget using the data property to provide your ThemeData. This ensures that the theme is applied to all widgets within the app.
MaterialApp( |
3. Custom Widgets: Create custom widgets that use the theme properties. This helps in reusing components throughout your app consistently.
class CustomButton extends StatelessWidget { |
4. Use Theme.of(context) Where Appropriate:
When styling widgets, prefer using Theme.of(context) to access the current theme. This ensures that your widgets are dynamically styled according to the theme.
Text( |
5. Text Themes and Typography:
Leverage the TextTheme and Typography classes provided by ThemeData for consistent text styling. Adjust font sizes, weights, and other text properties in a centralized manner.
ThemeData myTheme = ThemeData( |
6. Dark Mode and Light Mode:
Consider supporting both light and dark modes in your app by defining different themes. Flutter provides darkTheme property in MaterialApp for this purpose.
MaterialApp( |
7. Centralized Color Definitions:
If your app uses specific colors, define them in a centralized place to make it easier to update them later.
class AppColors { |
8. Separate Theme Configuration:
If your theme configuration becomes extensive, consider separating it into its own file or class to keep your main codebase clean.
class AppTheme { |
By following these best practices, you can create a centralized and well-organized theme for your Flutter app, making it easier to maintain and update the visual aspects of your application.
Ready to elevate your Flutter app design? Unlock the full potential of Flutter layouts with our professional Flutter developers.
(500+ Clients over 1000+ Projects)
Partner with YTII and build a team within 48 hours