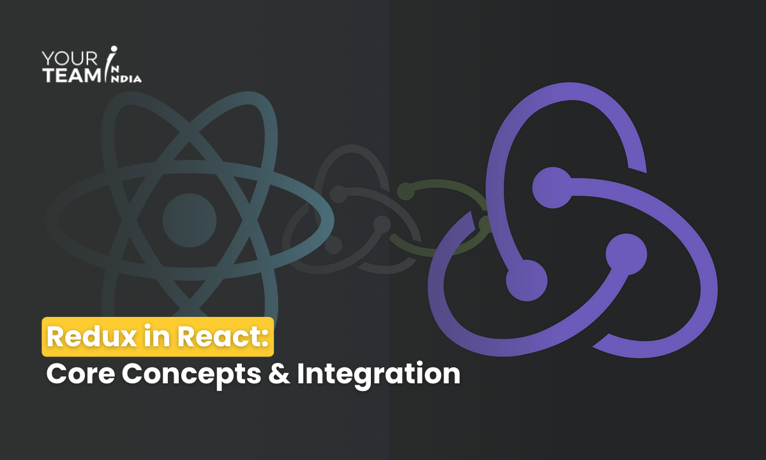
Quick Summary: Embark on a journey into the heart of React development with Redux, as we unravel its core concepts and seamless integration. Discover how this powerful state management library enhances your React applications, providing a structured and efficient way to manage and share state across components for a more streamlined development experience.
Introduction
Redux is a state management library that is frequently used in conjunction with React to manage an application's state reliably. It adheres to the Flux architecture tenets and is especially helpful for state management in expansive and intricate applications. Let's explore Redux's in-depth features within a React application.
Core Concept of Redux
- Store:
The store is a single source of truth for the state in your application. It holds the entire state tree of your application. You create the store using the 'createStore' function from Redux.
- Actions:
Simple JavaScript objects called actions are used to explain changes made to the application. A type property on them must indicate the 'type' of action being carried out.
- Reducers:
Reducers are simple functions that define how an action affects the state of the application. They return to the new state after accepting as arguments the prior state and an action.
- Dispatch:
The store uses a method called dispatch to send actions to the store. It is the sole means of inducing a shift in state.
- Subscribe:
A listener function can be added using the 'subscribe' method, which will trigger every time an action is dispatched and the state may have changed.
You can unsubscribe to stop listening to state changes.
React and Redux Integration
- Provider:
Using the 'Provider' component from 'react-redux,' the whole React application is wrapped, allowing all components to access the Redux store.
- Connect:
A React component is connected to the Redux store using the 'connect' function from 'react-redux'. "mapStateToProps" and "mapDispatchToProps" are the two arguments that are required.
- Redux Thunk(Middleware):
Redux middleware allows you to extend the store's abilities by intercepting dispatched actions. 'redux-thunk' is a popular middleware that enables the use of asynchronous actions.
Advantages
Redux is a state management library commonly used with React to manage the state of an application. Here are some advantages of using Redux in React:
- Centralized State Management: Redux centralizes the application state in a single store, making it easier to manage and debug the state of the entire application.
- Predictable State Changes: Redux follows a strict unidirectional data flow, making it easier to predict and understand how the state will change in response to actions.
- Debugging Capabilities: Redux provides powerful debugging tools such as time-travel debugging, which allows you to step forward and backward through state changes, making it easier to identify and fix issues.
- Ease of Testing: Since the state logic is separated from the React components, it becomes easier to test the state management logic independently, leading to more maintainable and testable code.
- Middleware Support: Redux allows the use of middleware, enabling you to extend its functionality with custom logic, such as logging, asynchronous operations, and more.
- Scalability: Redux is scalable for large and complex applications. It helps manage state in a structured way, making it easier to scale the application as it grows.
- State Persistence: Redux makes it simpler to implement features like undo/redo functionality or persisting the state to local storage.
- Community and Ecosystem: Redux has a large and active community, which means there are plenty of resources, plugins, and middleware available, making it easier to find solutions to common problems.
Disadvantages
Redux has a number of benefits, but it also has some drawbacks, especially in smaller or simpler applications. The following are some disadvantages of Redux in React:
- Boilerplate Code: Adding more boilerplate code for actions, action creators, reducers, and connecting components to the store is frequently necessary when implementing Redux in a React application. The codebase may become verbose as a result.
- Learning Curve: Redux adds new ideas and a different approach to state management, which may make the learning curve for developers—especially those who are not familiar with React or state management—more difficult.
- Verbosity: Redux, particularly in the case of basic applications, may result in more verbose code. Additional layers of abstraction are added by actions, action creators, and reducers; for smaller projects, these layers may not be needed.
- Overhead for Small Apps: Redux is better suited for larger and more complicated projects because the setup and maintenance costs for a store may be too great for small to medium-sized apps.
- Updates to Mutable States: Redux does not impose immutability, but it does promote it. In order to prevent unexpected behavior and bugs, developers must exercise discipline and refrain from directly changing the state.
- Component Coupling: Using the connect function to connect components to the Redux store may result in a higher degree of coupling between those components and the global state, which will hinder the reuse of those components in other contexts.
- Asynchronous Operations: Extra middleware, such as Redux Thunk, may be needed to handle asynchronous operations in Redux, such as API calls. This intricacy may work against you, particularly if you're just starting out.
- Possible Performance Impact: Depending on the situation, the overhead of maintaining a global state—especially if it's not done well—may exceed the performance benefits of centralizing state management in Redux.
Conclusion
Incorporating Redux into a React application offers a robust solution for managing complex states and enhancing predictability in state changes. Its centralized store facilitates a structured and scalable approach to state management, particularly beneficial in large applications where state interactions can become intricate.
The debugging capabilities, middleware support, and the ability to enforce a unidirectional data flow contribute to the maintainability and scalability of the codebase. However, the decision to use Redux should be carefully weighed against potential drawbacks, such as increased boilerplate code, a steeper learning curve, and potential verbosity, especially in smaller projects.
Ultimately, Redux is a powerful tool that shines in scenarios where a centralized and predictable state management system is crucial, but its adoption should be considered thoughtfully based on the specific needs and complexity of the application at hand.
Looking for the best talent to bring your web projects to life? Hire ReactJs developers to turn your vision into reality. Don't miss out on the opportunity to work with the top professionals in the field. Let's build something amazing together!