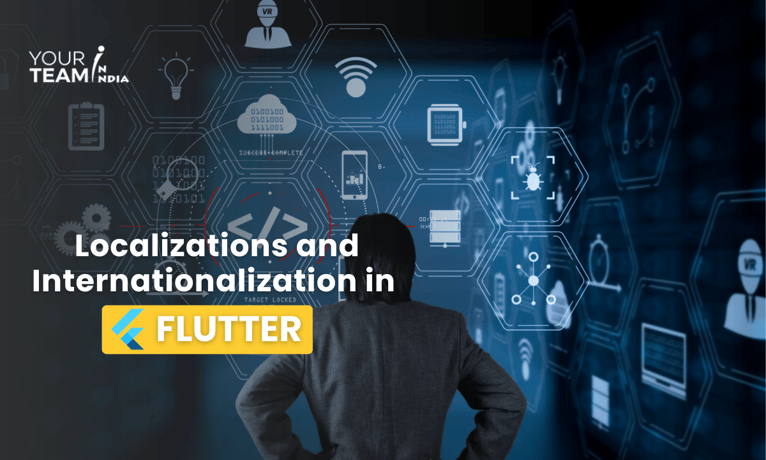
Quick Summary: In the global marketplace, app localization and internationalization are paramount. This article delves into how Flutter, a versatile mobile app development framework, empowers developers to adapt their apps for diverse international audiences. Discover essential techniques and best practices for creating multilingual and culturally relevant mobile experiences.
Localizations and Internationalization (i18n) are essential aspects of app development, enabling your Flutter app to adapt and provide content in different languages and regions. Flutter provides a powerful mechanism for implementing localization and internationalization in your app.
Here's a breakdown of the concepts and steps involved:-
- Localizations:
Localizations refer to the process of translating your app's user interface elements, such as text, labels, and messages, into different languages. Flutter uses the `Intl` package to handle localizations.
- Internationalization (i18n):
Internationalization encompasses the broader process of adapting your app to different languages, regions, and cultural norms. This includes not only translating text but also formatting dates, times, numbers, currencies, and more to match the user's preferred locale.
Key Takeaways
- Flutter's robust framework simplifies the process of localizing and internationalizing mobile apps, allowing for global reach.
- Effective use of Flutter's tools and libraries can streamline the translation and adaptation of app content for various languages and cultures.
- Tailoring user experiences to specific regions and languages is vital for engaging a broader audience and boosting app adoption.
- Understanding and implementing localization best practices is essential to ensure seamless functionality and user satisfaction in international markets.
Here are the steps to implement localization and internationalization in a Flutter app:
- Add Dependencies:
Start by adding the necessary dependencies to your `pubspec.yaml` file:
dependencies:
flutter:
sdk: flutter
flutter_localizations:
sdk: flutter
intl: ^your_version_here
- Create Localization Files:
Create JSON files containing translated strings for each supported language. For example, you might have `en.json` for English and `fr.json` for French. These files should be placed in a directory like `lib/l10n/`.
Sample `en.json`:
{
"greeting": "Hello",
"app_name": "My App"
}
- Configure Supported Locales:
In your app's main entry point (usually `main.dart`), configure the list of supported locales and set up the `MaterialApp` with the `LocalizationsDelegate`
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
localizationsDelegates: [
GlobalMaterialLocalizations.delegate,
GlobalWidgetsLocalizations.delegate,
// Add your custom delegate here
],
supportedLocales: [
const Locale('en', 'US'),
const Locale('fr', 'FR'),
// Add more locales as needed
],
home: MyHomePage(),
);
}
}
- Implement LocalizationsDelegate :
Create a custom `LocalizationsDelegate` to load and manage the localized resources:
class AppLocalizationsDelegate extends LocalizationsDelegate<AppLocalizations> {
@override
bool isSupported(Locale locale) {
return ['en', 'fr'].contains(locale.languageCode);
}
@override
Future<AppLocalizations> load(Locale locale) async {
AppLocalizations localizations = AppLocalizations(locale);
await localizations.load();
return localizations;
}
@override
bool shouldReload(covariant LocalizationsDelegate old) {
return false;
}
}
- Create Localizations Class:
Create a class that handles loading and retrieving localized strings:
class AppLocalizations {
final Locale locale;
AppLocalizations(this.locale);
static AppLocalizations of(BuildContext context) {
return Localizations.of<AppLocalizations>(context, AppLocalizations);
}
Map<String, String> _localizedStrings;
Future<void> load() async {
String jsonString = await rootBundle.loadString('lib/l10n/${locale.languageCode}.json');
Map<String, dynamic> jsonMap = json.decode(jsonString);
_localizedStrings = jsonMap.map((key, value) => MapEntry(key, value.toString()));
}
String translate(String key) {
return _localizedStrings[key] ?? key;
}
}
- Use Localized Strings:
In your app, use the `AppLocalizations` class to retrieve and display localized strings:
Text(AppLocalizations.of(context).translate('greeting'))
- Formatting Dates and Numbers:
For internationalization beyond text translation, you can use the `intl` package. For instance, to format dates and numbers:
DateFormat('dd/MM/yyyy').format(DateTime.now())
NumberFormat.currency(locale: 'en_US', symbol: '\$').format(42.5)
- Change App Locale:
To change the app's locale dynamically, you can use a package like `provider` to manage the state and update the locale accordingly.
Remember that internationalization is a complex topic that goes beyond simple translation. Cultural differences, number and date formats, and other regional aspects should be considered for a complete internationalization strategy.
By following these steps, you'll be able to create a Flutter app that supports multiple languages and regions, providing a seamless experience to users around the world.
Internationalization vs. Localization
Internationalization (often abbreviated as "i18n") and localization (often abbreviated as "l10n") are two closely related concepts in the context of software development, particularly in creating applications, websites, or software that can be used by people from different regions and cultures.
1. Internationalization (i18n):
Internationalization is the process of designing and developing software in a way that makes it easy to adapt to various languages, regions, and cultures without changing the core codebase. The goal of internationalization is to create a foundation that allows for seamless translation and adaptation of content, user interfaces, and other elements to suit the preferences and requirements of different locales.
Key aspects of internationalization include:
- Design and Architecture: Designing the software's architecture and user interface in a way that separates content from code logic, making it easier to replace or adapt text and graphical elements for different languages and cultures.
- Character Encoding: Ensuring that the software supports Unicode or other appropriate character encodings to handle various languages and scripts.
- Date and Time Formats: Adapting date, time, and numeric formats to match the conventions of different regions.
- User Interface Layout: Allowing for dynamic adjustments of user interface elements to accommodate variations in text length due to translation.
- Cultural Considerations: Taking into account differences in symbols, colors, and cultural norms that might affect how the software is perceived and used in different regions.
2. Localization (l10n):
Localization is the process of adapting a software application or content to a specific locale or target market. This involves translating and customizing elements such as text, images, formatting, and user interface components to align with the linguistic and cultural preferences of a particular audience. Localization goes beyond just language translation; it also includes adapting elements like date formats, currency symbols, and other cultural nuances.
Key aspects of localization include:
- Translation: Converting text, labels, and messages from the source language into the target language.
- Cultural Adaptation: Adjusting content to suit local cultural norms, values, and practices.
- Date and Time Formats: Changing date, time, and numeric formats to match those commonly used in the target region.
- Currency and Measurement Units: Converting currency symbols and measurement units to those relevant to the target market.
- Images and Graphics: Replacing images or graphics that may not be suitable or relevant for the target culture.
- Legal and Regulatory Compliance: Ensuring that the software complies with legal requirements and regulations of the target region.
In essence, internationalization lays the groundwork for making a software application adaptable to different locales, while localization involves the actual customization and translation of the software for specific regions. Both concepts are crucial for creating user-friendly and culturally appropriate software experiences for a global audience.
Conclusion:-
In conclusion, implementing localization and internationalization in Flutter is essential for creating a user-friendly and globally accessible app. While localizations focus on translating text and labels into different languages, internationalization goes beyond translation to include adapting the app's formatting, date and number conventions, and other cultural aspects to match the preferences of users from diverse regions.
By following the steps outlined earlier, you can ensure that your Flutter app provides a seamless experience for users around the world, regardless of their language or location. Properly localized and internationalized apps not only enhance user engagement but also demonstrate a commitment to inclusivity and user satisfaction on a global scale.
Unlock the potential of Flutter and elevate your mobile app projects with our team of expert Flutter developers. Hire our Flutter Developers to bring your app idea to life.