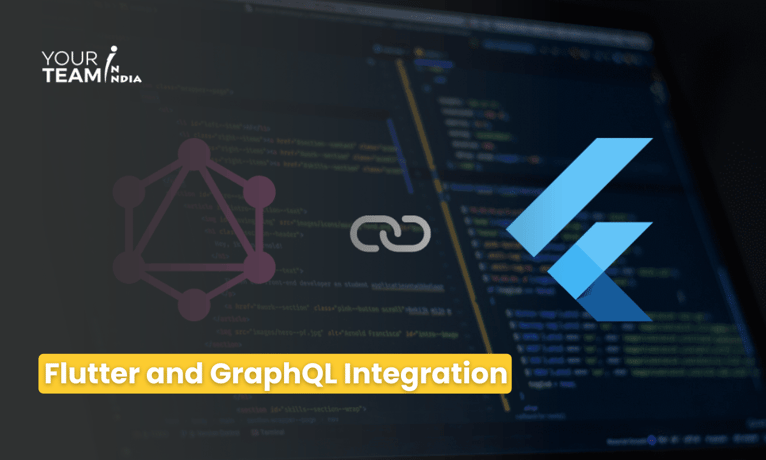
Quick Summary: This comprehensive guide dives into the integration of Flutter and GraphQL. It explores how to leverage GraphQL to efficiently query and manage data in Flutter apps, enabling seamless communication with backend services and enhancing user experiences.
Introduction
- The evolution of data fetching in mobile apps, from REST to GraphQL.
- Overview of GraphQL as a query language for APIs and its benefits over traditional REST.
- How Flutter facilitates seamless integration with GraphQL for efficient data fetching.
Getting Started with GraphQL in Flutter
Introduction to GraphQL
- Explanation of GraphQL as a query language for APIs.
- Key features, such as query flexibility and reduced over-fetching.
- Code snippets introducing the basics of GraphQL queries.
// Example GraphQL query in Flutter |
Setting Up GraphQL in Flutter Project
- Overview of packages for GraphQL integration in Flutter (e.g., graphql_flutter).
- Step-by-step guide on setting up GraphQL dependencies.
- Code examples for initializing a GraphQL client in Flutter.
|
// Initializing a GraphQL client in Flutter |
Executing GraphQL Queries in Flutter
- Fetching Data with Queries
- Implementation of basic GraphQL queries for data retrieval.
- Code examples demonstrating how to send queries and handle responses.
Query( |
Passing Variables in GraphQL Queries
- Explanation of the importance of variables in GraphQL queries.
- Code snippets illustrating how to pass variables in Flutter GraphQL queries.
// Example GraphQL query with variables |
GraphQL Mutations and Subscriptions in Flutter
- Executing Mutations
- Introduction to GraphQL mutations for data modification.
- Code examples demonstrating how to execute mutations in Flutter.
Mutation( |
Real-Time Updates with Subscriptions
- Explanation of GraphQL subscriptions for real-time data updates.
- Code snippets illustrating how to implement GraphQL subscriptions in Flutter.
Subscription( |
Handling GraphQL Errors and Optimizing Queries
- Error Handling in GraphQL
- Strategies for handling errors in GraphQL queries and mutations.
- Code examples demonstrating error handling techniques in Flutter.
Query( |
- Optimizing GraphQL Queries
- Best practices for optimizing GraphQL queries in Flutter apps.
- Techniques such as batching and caching for improved performance.
- Code snippets showcasing query optimization strategies.
Conclusion
- Recap of the advantages of using GraphQL in Flutter for efficient data fetching.
- Developers should be encouraged to explore GraphQL's capabilities for a more flexible and streamlined data layer.
- Reminders about the importance of handling errors and optimizing queries for optimal app performance.
Hire Flutter developers to elevate your Flutter app design. Unlock the full potential of Flutter layouts with our professional Flutter developers.